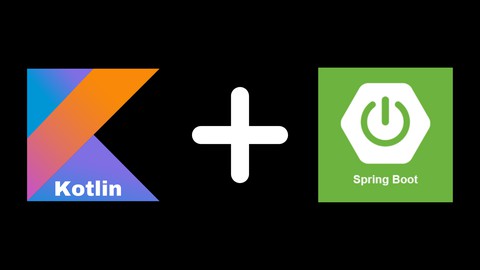
Build RESTFUL APIs using Kotlin and Spring Boot
Build RESTFUL APIs using Kotlin and Spring Boot, available at $74.99, has an average rating of 4.48, with 93 lectures, based on 903 reviews, and has 6636 subscribers.
You will learn about Kotlin Programming Language and its benefits Write Code using Kotlin Programming Language Building Applications using Kotlin Build RestFul Services using SpringBoot and Kotlin Test Kotlin using JUnit5 Kotlin and Java Interoperability Integrate Spring Data JPA with Kotlin Integration testing DB Layer using TestContainers & JUnit5 Unit Testing Functions calls using Mockk Library This course is ideal for individuals who are Developers who are interested in learning Kotlin Programming language or Developers who are interested in building applications using Kotlin and SpringBoot or Java developers who would like to learn Kotlin Programming Language It is particularly useful for Developers who are interested in learning Kotlin Programming language or Developers who are interested in building applications using Kotlin and SpringBoot or Java developers who would like to learn Kotlin Programming Language.
Enroll now: Build RESTFUL APIs using Kotlin and Spring Boot
Summary
Title: Build RESTFUL APIs using Kotlin and Spring Boot
Price: $74.99
Average Rating: 4.48
Number of Lectures: 93
Number of Published Lectures: 93
Number of Curriculum Items: 97
Number of Published Curriculum Objects: 97
Original Price: $89.99
Quality Status: approved
Status: Live
What You Will Learn
- Kotlin Programming Language and its benefits
- Write Code using Kotlin Programming Language
- Building Applications using Kotlin
- Build RestFul Services using SpringBoot and Kotlin
- Test Kotlin using JUnit5
- Kotlin and Java Interoperability
- Integrate Spring Data JPA with Kotlin
- Integration testing DB Layer using TestContainers & JUnit5
- Unit Testing Functions calls using Mockk Library
Who Should Attend
- Developers who are interested in learning Kotlin Programming language
- Developers who are interested in building applications using Kotlin and SpringBoot
- Java developers who would like to learn Kotlin Programming Language
Target Audiences
- Developers who are interested in learning Kotlin Programming language
- Developers who are interested in building applications using Kotlin and SpringBoot
- Java developers who would like to learn Kotlin Programming Language
Kotlin is the Modern, concise and safe programming languageand is one of the popular JVM language in this day and age.
It’s also interoperable with Java and other languages, and provides many ways to reuse code between multiple platforms for productive programming.
This course will focus on using Kotlin for Server-Side Developmentusing SpringBootframework. This is a pure hands-on oriented course which covers these two topics:
-
Covers Kotlin Fundamentals thats necessary for Java Developers
-
Build RestFul APIsusing SpringBoot and Kotlin
Section 1: Getting Started With the Course
-
This section covers the course objectives and the prerequisites that are needed to make the most out of this course.
Section 2:Getting Started with Kotlin Programming Language
-
In this section, I will introduce you to Kotlin Programming Language and why its a powerful language for enterprise development.
-
Introduction to Kotlin
-
How Kotlin Works with the JVM?
-
Section 3: Kotlin Fundamentals
-
In this section, we will explore the fundamentals of Kotlin.
-
val & var variables in Kotlin
-
Basic Types – Int, Long, Double, String
-
Conditionals – If and when block
-
Ranges , Loops
-
while & do-While
-
break, labels and return
-
Section 4: Functions in Kotlin
-
In this section, we will learn about functions in Kotlin and different ways of declaring and using them
-
Defining and Invoking Functions
-
Default Value Parameters & Named Arguments
-
Top-Level Functions and Top-level Properties
-
Section 5: Classes, Interfaces and Inheritance
-
In this section, we will learn about classes, inheritance and interfaces in detail.
-
Introduction to class – Creating a class and objects
-
Primary Constructors
-
Secondary Constructors
-
initializer code using init block
-
Data Classes
-
Custom Getters and Setters
-
Inheritance – Extending Classes
-
Inheritance – Override Functions, Variables
-
object keyword for creating instance of the class
-
companion object Keyword
-
Interfaces
-
Interfaces – Handling Conflicting Functions
-
Interfaces – Defining and Overrding Variables
-
Visibility Modifiers
-
Type Checking, Casting and Smart Cast
-
Enum class
-
Section 6: Nulls in Kotlin
-
In this section, we will learn about handling nulls in Kotlin
-
Nullable & Non-Nullable types in Kotlin
-
Safe Call(?) , Elvis Operator(?:) & Non Null Assertion(!!) to deal with Null Values
-
Invoking or assigning a Nullable Type to a Non-Nullable Type
-
Section 7: Collections, Arrays & Lamda Expressions
-
In this section, I will introduce you to collections, arrays and lambda expressions in Kotlin
-
Introduction to Collections
-
Introduction to Lamda Expressions
-
Lambdas and Higher Order Functions
-
Filter Operations on Kotlin Collections
-
Map Operations on Kotlin Collections
-
FlatMap Operations in Collections
-
Working With HashMaps
-
Lazy Evaluation of Collections using Sequences
-
Nullability in Collections
-
Section 8 : Exceptions In Kotlin
-
In this section, I will cover the exceptions in kotlin and the techniques to handle them.
-
Handling Exceptions using try-catch
-
Section 9 : Scope Functions
-
In this section, I will introduce you all to scope functions in Kotlin and its usage.
-
Introduction to Scope Functions
-
apply & also Scope Function
-
let Scope Function
-
with & run Scope Function
-
Section 10 : Getting Started with Kotlin and Spring Boot
-
In this section, I will explain the overview of the app we are going to build and build a very simple API.
-
Overview of the app & Project Setup
-
Build a Simple Endpoint – Greeting Controller
-
Constructor Injection in Spring
-
Setting up different profiles in Spring Boot
-
Set up Logging in Kotlin
-
Section 11 : Integration/Unit Testing using Junit 5
-
In this section, I will code and explain about the techniques to write different types of test cases using spring boot and Kotlin.
-
Introduction to Automated Tests & Setting up JUnit5
-
Integration Test for Controller
-
Unit Test for Controller – Using the Mockk Mocking library
-
Section 12 : Build the Course Catalog Service
-
In this section, we will build the Course Catalog Service to manage the Courses
-
Set up the Course Entity & CourseDTO
-
Create CourseRepository & Configure JPA in application.yml file
-
Build the POST Endpoint for adding new Course I
-
ntegration test for the POST endpoint using JUnit5
-
Build the Get Endpoint to retrieve all Courses
-
Integration test for the GET endpoint to retrieve all the courses
-
Build the Update Endpoint to update a Course
-
Integration test for the PUT endpoint using JUnit5
-
Build the DELETE endpoint to delete a Course
-
Section 13: Unit Testing Controller layer (Web Tier)
-
In this section, we will code and learn about how to write unit tests for the controller
-
Setting up the Unit Test for the CourseController
-
Unit test for the Post Endpoint in CourseController
-
Unit test for the GET Endpoint in CourseController
-
Unit test for the PUT Endpoint in CourseController
-
Unit test for the DELETE Endpoint in CourseController
-
Section 14 : Bean Validation using Validators and ControllerAdvice
-
In this section, we will code and learn the different techniques to apply bean validations and handle exceptions using the ControllerAdvice Pattern
-
Name and Category as Mandatory using @NotBlank Annotation
-
Implement Custom Error Handling using ControllerAdvice pattern
-
Handle Global RuntimeException using ControllerAdvice Pattern
-
Section 15 : Custom JPA queries using Spring Data JPA and DB Layer testing using @DataJpaTest
-
In this lecture, we will learn about the techniques to write custom JPA queries and the techniques to test the DB layer using the DataJpaTest
-
Retrieve Courses By Name using JPA Query Creation Function
-
Retrieve Courses By Name using Native SQL query
-
Testing Mutliple sets of Data using @Parameterized test
-
Section 16: GET Endpoint to retrieve Courses By Name using @RequestParam
-
In this section, we will code and learn about the usage of RequestParam in the controller endpoint.
-
Use existing GET endpoint to retrieve Courses by Name
-
Write Integration test to retrieve course by Name
-
Section 17 : Entity RelationShips using Spring Data JPA
-
In this section, I will explain the technique to express the relationships in JPA using Entity and Data classes in Kotlin
-
Adding Instructor Entity in to the Course Catalog Service
-
Adding the relationship in the Entity Class
-
Instructor Controller to Manage Instructor Data
-
Update CourseService to validate Instructor Data
-
Fix the CourseController Integration Tests
-
Fix the CourseController Unit Tests
-
Section 18 : Integrating with Postgres DB
-
In this section, we will code and learn to integrate the postgres DB in to the course catalog service.
-
Setting up the Postgres DB and App to interact with Postgres
-
Test the app with Postgres DB
-
Section 19 : Integration Testing using TestContainers
-
In this section, we will code and learn to integrate the testcontainers to run integration test.
-
Setting Up TestContainers for the Integration Test
-
Configure @DataJpaTest with TestContainers
-
Section 20 : Java & Kotlin Interoperability
-
In this section, we will code and learn about the interoperability between Java and Kotlin.
-
Invoking Kotlin Code from Java Class
-
Invoking Java Code from Kotlin
-
Useful JVM annotations in Kotlin
-
By the end of this course, you will be comfortable writing code using the Koltin Programming language and Build RestFuL APIs using SpringBoot and Kotlin.
Course Curriculum
Chapter 1: Getting Started with the Course
Lecture 1: Course Introduction
Lecture 2: Prerequisites
Chapter 2: Course Slides & Source Code
Lecture 1: Course Slides
Lecture 2: Source Code
Chapter 3: Getting Started with Kotlin Programming Language
Lecture 1: Introduction to Kotlin
Lecture 2: How Kotlin Works with the JVM?
Chapter 4: Kotlin Fundamentals
Lecture 1: Project Setup
Lecture 2: Hello Kotlin!
Lecture 3: val & var variables in Kotlin
Lecture 4: Basic Types – Int, Long, Double, String
Lecture 5: Conditionals – If and when block
Lecture 6: Ranges & Loops
Lecture 7: while & do-While
Lecture 8: break, labels and return
Chapter 5: Functions in Kotlin
Lecture 1: Defining and Invoking Functions
Lecture 2: Default Value Parameters & Named Arguments
Lecture 3: Top-Level Functions and Top-level Properties
Chapter 6: Classes, Interfaces and Inheritance
Lecture 1: Introduction to class – Creating a class and objects
Lecture 2: Primary Constructors
Lecture 3: Secondary Constructors
Lecture 4: initializer code using init block
Lecture 5: Data Classes
Lecture 6: Custom Getters and Setters
Lecture 7: Inheritance – Extending Classes
Lecture 8: Inheritance – Override Functions, Variables
Lecture 9: object keyword for creating instance of the class
Lecture 10: companion object Keyword
Lecture 11: Interfaces
Lecture 12: Interfaces – Handling Conflicting Functions
Lecture 13: Interfaces – Defining and Overrding Variables
Lecture 14: Visibility Modifiers
Lecture 15: Type Checking, Casting and Smart Cast
Lecture 16: Enum class
Chapter 7: Nulls in Kotlin
Lecture 1: Nullable & Non-Nullable types in Kotlin
Lecture 2: Safe Call(?) ,Elvis Operator(?:),Non Null Assertion(!!) to deal with Null Values
Lecture 3: Invoking or assigning a Nullable Type to a Non-Nullable Type
Chapter 8: Collections, Arrays & Lamda Expressions
Lecture 1: Introduction to Collections
Lecture 2: Introduction to Lamda Expressions
Lecture 3: Lambdas and Higher Order Functions
Lecture 4: Filter Operations on Kotlin Collections
Lecture 5: Map Operations on Kotlin Collections
Lecture 6: FlatMap Operations in Collections
Lecture 7: Working With HashMaps
Lecture 8: Lazy Evaluation of Collections using Sequences
Lecture 9: Nullability in Collections
Chapter 9: Exceptions In Kotlin
Lecture 1: Handling Exceptions using try-catch
Chapter 10: Scope Functions
Lecture 1: Introduction to Scope Functions
Lecture 2: apply & also Scope Function
Lecture 3: let Scope Function
Lecture 4: with & run Scope Function
Chapter 11: Getting Started with Kotlin and Spring Boot
Lecture 1: Overview of the app & Project Setup
Lecture 2: Build a Simple Endpoint – Greeting Controller
Lecture 3: Constructor Injection in Spring
Lecture 4: Setting up different profiles in Spring Boot
Lecture 5: Set up Logging in Kotlin
Chapter 12: Integration/Unit Testing using Junit5
Lecture 1: Introduction to Automated Tests & Setting up JUnit5
Lecture 2: Integration Test for Controller
Lecture 3: Unit Test for Controller – Using the Mockk Mocking library
Chapter 13: Build the Course Catalog Service
Lecture 1: Set up the Course Entity & CourseDTO
Lecture 2: Create CourseRepository & Configure JPA in application.yml file
Lecture 3: Build the POST Endpoint for adding new Course
Lecture 4: Integration test for the POST endpoint using JUnit5
Lecture 5: Build the Get Endpoint to retrieve all Courses
Lecture 6: Integration test for the GET endpoint to retrieve all the courses
Lecture 7: Build the Update Endpoint to update a Course
Lecture 8: Integration test for the PUT endpoint using JUnit5
Lecture 9: Build the DELETE endpoint to delete a Course
Chapter 14: Unit Testing Controller layer (Web Tier)
Lecture 1: Setting up the Unit Test for the CourseController
Lecture 2: Unit test for the Post Endpoint in CourseController
Lecture 3: Unit test for the GET Endpoint in CourseController
Lecture 4: Unit test for the PUT Endpoint in CourseController
Lecture 5: Unit test for the DELETE Endpoint in CourseController
Chapter 15: Bean Validation using Validators and ControllerAdvice
Lecture 1: Name and Category as Mandatory using @NotBlank Annotation
Lecture 2: Implement Custom Error Handling using ControllerAdvice pattern
Lecture 3: Handle Global RuntimeException using ControllerAdvice Pattern
Chapter 16: Custom JPA queries using Spring Data JPA and DB Layer testing using @DataJpaTest
Lecture 1: Retrieve Courses By Name using JPA Query Creation Function
Lecture 2: Retrieve Courses By Name using Native SQL query
Lecture 3: Testing Mutliple sets of Data using @Parameterized test
Chapter 17: GET Endpoint to retrieve Courses By Name using @RequestParam
Lecture 1: Use existing GET endpoint to retrieve Courses by Name
Lecture 2: Write Integration test to retrieve course by Name
Chapter 18: Entity RelationShips using Spring Data JPA
Lecture 1: Adding Instructor Entity in to the Course Catalog Service
Instructors
-
Pragmatic Code School
Technology Enthusiast, Online Instructor
Rating Distribution
- 1 stars: 11 votes
- 2 stars: 21 votes
- 3 stars: 80 votes
- 4 stars: 313 votes
- 5 stars: 478 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Ultimate all royalty free contents for youtubers or bloggers
- Complete WhatsApp Marketing Course
- YouTube 1M Subscribers Guide – Proven ways for YouTube Subs
- Sales Funnel Blueprint For Beginners
- Instagram Business Marketing Game Plan 10k+ Paying Customers
- Earning Online Made Easy: CPA Marketing for Beginners
- MarketHealth Mastery: Dominating Sales in 90 Days
- How to Use Instagram Video for Business and Building a Brand
- YouTube Piggyback Method – Unlimited Cheap Traffic
- Learning Search Engine Optimization (SEO) from Scratch
- Rock Your Newsletter
- Google My Business – Complete Listing Optimization Training
- Workflow in Salesforce
- Mastering Invideo AI – A Complete Guide to Text-to-Video
- Ultimate Content Marketing Workshop ⎢Certificate Course 2024
- Copywriting For Beginners Course- Start Making Money Today
- Mastering Facebook Ads: From Beginner to Expert
- Content Creation with Midjourney
- Search Engine Marketing (SEM): #1 Step-by-Step Tutorial
- The Sales Funnel Cheat Sheet Collection – 4 Courses In 1