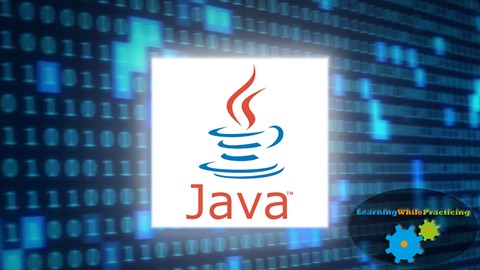
Java: a COMPLETE tutorial from ZERO to JDBC
Java: a COMPLETE tutorial from ZERO to JDBC, available at $64.99, has an average rating of 4.9, with 154 lectures, based on 84 reviews, and has 366 subscribers.
You will learn about Java programming language Java Development Kit (JDK) GUI programming with Java Java Database Connectivity (JDBC) This course is ideal for individuals who are Students from any level who want to learn Java programming It is particularly useful for Students from any level who want to learn Java programming.
Enroll now: Java: a COMPLETE tutorial from ZERO to JDBC
Summary
Title: Java: a COMPLETE tutorial from ZERO to JDBC
Price: $64.99
Average Rating: 4.9
Number of Lectures: 154
Number of Published Lectures: 154
Number of Curriculum Items: 154
Number of Published Curriculum Objects: 154
Original Price: β¬219.99
Quality Status: approved
Status: Live
What You Will Learn
- Java programming language
- Java Development Kit (JDK)
- GUI programming with Java
- Java Database Connectivity (JDBC)
Who Should Attend
- Students from any level who want to learn Java programming
Target Audiences
- Students from any level who want to learn Java programming
Java is one of the most prominent programming languages today due its power and versatility.
Our concept at LearningWhilePracticing is to guide you to be operational right away. We help you uncover new skills through practice, instead of going over a boring class where you would not be grasping the concepts. Practices makes perfect!
In this course, you will be guided by Oracle certified Java expert Mr Lawrence Decamora, from start to finish.
NO JAVA KNOWLEDGE IS PREVIOUSLY REQUIRED!
This Java tutorial is made up of 14 sections:
-
Section 0: Setting up your Java Development Kit
-
Downloading, Installing and Configuring your JDK
-
Windows OS
-
Mac OS
-
-
Setting up your IDE
-
Eclipse
-
NetBeans
-
-
-
Section 1: Your First Java Cup
-
How to write your first Java Program –> HelloWorld.java
-
How to save, compile and run your first Java Program
-
How to debug a compilation error
-
The Parts of your Java Program
-
class
-
the main method
-
the System.out.println() method
-
the (+) operator
-
the ‘n’ and ‘t’ characters
-
-
Commonly encountered errors:
-
Misspelled public class name vs misspelled filename
-
Misspelled keywords and method names
-
Incorrect location of your file or your present working directory.
-
-
-
Section 2: Difference between a Class and an Object
-
How to create an object
-
Constructors
-
instance variables
-
instance methods
-
-
How to use / test an object.
-
Encapsulation (Data Hiding)
-
Java API Documentation
-
The import Statement
-
-
Section 3: Introducing the use of an Integrated Development Environment (IDE)
-
Eclipse
-
Netbeans
-
IntelliJ
-
Creating our first Netbeans Project
-
Comments in Java
-
single line comments
-
multi line comments
-
java doc comments
-
-
The Semi-colon;
-
The { } blocks
-
Whitespaces
-
The import Statement
-
The package Statement
-
Java Data Types
-
Reference Data Types (To be discussed in Section 5)
-
Primitive Data Types
-
byte
-
short
-
int
-
long
-
float
-
double
-
char
-
boolean
-
-
-
The Scanner object.
-
The nextXxx() methods
-
-
-
Section 4: Operators and Control Structures
-
Operators
-
Casting
-
Increment / Decrement (++ / –)
-
Mathematical (*, /, %, +, -)
-
Relational (<, <=, >, >=, ==, !=)
-
Logical Operators (&, |, ^)
-
Short-Circuit Operators (&&, ||)
-
Ternary Operators (? π
-
Assignment and Short-Hand Operators
-
-
Control Structures
-
if-else
-
switch-case
-
while
-
do while
-
for loop
-
nested loops
-
break and continue statements
-
labeled break and labeled continue statements
-
-
-
Section 5: The Reference Data Types
-
Primitive Data Types vs Reference Data Type
-
User Defined Classes –> Reference Data Types
-
Assigning References to Variables
-
Pass by Value and Local Variable Scopes
-
The this Reference
-
-
Section 6: Arrays and Strings
-
Array Creation and Initialization
-
Array Limits (.length)
-
The Enhanced for loop
-
Copying Arrays
-
Command-Line Arguments
-
The parse Methods
-
-
Array of Arrays (Two-dimensional Arrays)
-
Non-Rectangular Arrays
-
String, StringBuffer and StringBuilder
-
-
Section 7: Inheritance and Polymorphism
-
Inheritance: Classes, Superclasses, and Subclasses
-
Single Inheritance
-
The βis-aβ relationship
-
Java Access Modifiers
-
Method Overriding
-
Rules in Overriding a Method
-
The super keyword
-
Polymorphism
-
Virtual Method Invocation and Heterogeneous Array
-
Polymorphic Arguments
-
The instanceof operator
-
Casting of Objects
-
Overloading Methods
-
Rules for Method Overloading
-
Inheritance and Constructors
-
Overloading Constructors
-
The Object class (equals(), hashCode() and toString() methods)
-
The static keyword
-
How do we access / call a static variable or a static method?
-
The Math and System Classes
-
The static imports
-
-
Section 8: Other Class Features
-
The Wrapper Classes
-
The final keyword
-
The enum keyword
-
The abstract keyword
-
Java Interfaces
-
The Interface default methods
-
The Interface static methods
-
-
The Functional Interface and the Lambda (->) Operator
-
-
Section 9: Exceptions and Assertions
-
The Exception and The Error class
-
Why do we need to have Exception Handling?
-
Sample Exceptions
-
Javaβs Approach, the Call Stack Mechanism
-
The five keywords used for Exception handling or Exception declaration
-
General Syntax of an exception – handling block
-
The try β catch block
-
The finally block
-
The Exception Hierarchy in Java
-
Multiple Exceptions in a catch Block
-
The parameterized try block
-
The Handle-or-Declare rule
-
The throws keyword
-
Rules on Overriding Methods and Exceptions
-
Creating your own Exception objects
-
Assertion Checks
-
-
Section 10: IO and FileIO
-
How to accept inputs using:
-
The Scanner Class
-
The BufferedReader and InputStreamReader Classes
-
-
How to format an output.
-
The File class.
-
How to read and write inputs from and to a File.
-
-
Section 11: The Collection and Generics Framework
-
The Collection Interface
-
The Set Interface
-
The List Interface
-
The Map Interface
-
The Iterator Interface
-
The Generics Framework
-
Creating your own Set of Collection objects
-
Sorting your Collection
-
-
Section 12: Building a GUI Based Desktop Application
-
The AWT package
-
Components, Containers, and Layout Managers.
-
Using selected layout managers to achieve desired GUI layout.
-
Add components to a containers
-
Demonstrate how complex layout manager and nested layout managers works.
-
Define what events, event sources, and event handlers are.
-
Event handling techniques
-
Write code to handle events that occur in a GUI
-
Describe the five (5) ways on how to implement event handling technique.
-
Converting the AWT code to a Swing application
-
Packaging a JAR file for application deployment
-
-
Section 13: Introduction to JDBC
-
β Introduction to Database Concepts
-
β How to Create your first DB Schema
-
– Basic SQL Statements
-
– SELECT
-
– UPDATE
-
– DELETE
-
– INSERT
-
-
– What is JDBC?
-
– The Statement Interface
-
– The PreparedStatement Interface
-
-
Each section contains its ressources files (codes and notes used by the instructor)
Whether you’re a complete beginner or already got knowledge in Java, this course is for you! Happy Coding!
Course Curriculum
Chapter 1: Setting up your Java Development Kit
Lecture 1: Section0_01 – Section Introduction
Lecture 2: Section0_02 – Setting up your JDK on your Mac
Lecture 3: Section0_03 – Setting up your NetBeans on your Mac
Lecture 4: Section0_04 – Testing your JDK and NetBeans on your Mac
Lecture 5: Section0_05 – Setting up your JDK on your Windows Machine
Lecture 6: Section0_06 – Setting up and Test your NetBeans on your Windows Machine
Chapter 2: Your First Java Cup
Lecture 1: Section1_01 – Your First Java Program – HelloWorld
Lecture 2: Section1_02 – Commonly Encountered Errors
Lecture 3: Section1_03 – Parts of Your Java Program
Chapter 3: Difference between a Class and an Object
Lecture 1: Section2_01 – Difference Between a Class and Objects
Lecture 2: Section2_02 – The Person and TestPerson Classes
Lecture 3: Section2_03 – Constructors
Lecture 4: Section2_04 – Methods
Lecture 5: Section2_05 – Encapsulation (aka Data Hiding)
Lecture 6: Section2_06 – The Java API Documentation
Lecture 7: Section2_07 – The import statement
Chapter 4: Introducing the use of an Integrated Development Environment (IDE)
Lecture 1: Section3_01 – Integrated Development Environment (IDE)
Lecture 2: Section3_02 – Your First NetBeans Project
Lecture 3: Section3_03 – Comments, Semi-colon, Blocks and Whitespaces
Lecture 4: Section3_04 – The import and the package statements
Lecture 5: Section3_05 – Java Data Types
Lecture 6: Section3_06 – The Scanner object and the nextXxx() Methods
Chapter 5: Operators and Control Structures
Lecture 1: Section4_01 – Section Introduction
Lecture 2: Section4_02 – Data Type Casting, part 1
Lecture 3: Section4_03 – Data Type Casting, part 2
Lecture 4: Section4_04 – Data Type Casting, part 3
Lecture 5: Section4_05 – The Increment and Decrement Operators
Lecture 6: Section4_06 – The Mathematical Operators
Lecture 7: Section4_07 – The Relational Operators
Lecture 8: Section4_08 – The Logical Operators and the Short-Circuit Operators, part 1
Lecture 9: Section4_09 – The Logical Operators and the Short-Circuit Operators, part 2
Lecture 10: Section4_10 – The Ternary Operator
Lecture 11: Section4_11p – The Ternary Operator Sample Problem – Odd / Even
Lecture 12: Section4_11s – The Ternary Operator Solution – Odd / Even
Lecture 13: Section4_12 – The Assignment and Short-Hand Operators
Lecture 14: Section4_13 – The if-else condition
Lecture 15: Section4_14 – The if-else-if ladder and age problem
Lecture 16: Section4_15 – The age problem solution
Lecture 17: Section4_16 – The nested if
Lecture 18: Section4_17 – The switch-case statement
Lecture 19: Section4_18p – The month to word problem
Lecture 20: Section4_18s – The month to word solution
Lecture 21: Section4_19p – The taxi fare problem
Lecture 22: Section4_19s – The taxi fare solution
Lecture 23: Section4_20 – The while and the do-while loops, part 1
Lecture 24: Section4_21 – The while and the do-while loops, part 2
Lecture 25: Section4_22 – Infinite Loops
Lecture 26: Section4_23p – Print all numbers from the smaller to the larger number problem
Lecture 27: Section4_23s – Print all numbers from the smaller to the larger number solution
Lecture 28: Section4_24 – The for loop, part 1
Lecture 29: Section4_25 – The for loop, part 2
Lecture 30: Section4_26p – Print the odd numbers from 1 to 10 problem
Lecture 31: Section4_26s – Print the odd numbers from 1 to 10 solution
Lecture 32: Section4_27 – Nested loops
Lecture 33: Section4_28p – Print the Multiplication Table Problem
Lecture 34: Section4_28s – Print the Multiplication Table Solution
Lecture 35: Section4_29 – The break and continue statements
Lecture 36: Section4_30 – Labelled breaks and labelled continues
Chapter 6: The Reference Data Types
Lecture 1: Section5_01 – The Reference Data Types
Lecture 2: Section5_02 – User Defined Classes
Lecture 3: Section5_03 – Assigning a Reference Value to a Variable
Lecture 4: Section5_04 – Pass by Value and Local Variable Scopes
Lecture 5: Section5_05p – The String Problem
Lecture 6: Section5_05s – The String Solution
Lecture 7: Section5_06 – The 'this' keyword, part 1
Lecture 8: Section5_07 – The 'this' keyword, part 2
Chapter 7: Arrays and Strings
Lecture 1: Section6_01 – Section Introduction, Arrays and Strings
Lecture 2: Section6_02 – Array Creation and Initialization, part 1
Lecture 3: Section6_03 – Array Creation and Initialization, part 2
Lecture 4: Section6_04 – Array Limits, the .length attribute
Lecture 5: Section6_05 – Sample Array Problem and Solution
Lecture 6: Section6_06 – The Enhanced for loop
Lecture 7: Section6_07 – Copying of Arrays
Lecture 8: Section6_08 – Command Line Arguments and the parseXxx Methods
Lecture 9: Section6_09 – The Two Dimensional Arrays, aka an Array of Arrays
Lecture 10: Section6_10p – The Multiplication Table Problem
Lecture 11: Section6_10s – The Multiplication Table Solution
Lecture 12: Section6_11 – The Non-Rectangular Arrays
Lecture 13: Section6_12p – The Calendar Problem
Lecture 14: Section6_12s – The Calendar Solution
Lecture 15: Section6_13 – The String, StringBuffer and StringBuilder, part 1
Lecture 16: Section6_14 – The String, StringBuffer and StringBuilder, part 2
Lecture 17: Section6_15p – The Palindrome Problem
Lecture 18: Section6_15s – The Palindrome Solution
Chapter 8: Inheritance and Polymorphism
Lecture 1: Section7_01 – Inheritance Concepts
Lecture 2: Section7_02 – Java Access Modifiers
Lecture 3: Section7_03 – Method Overriding
Lecture 4: Section7_04 – The 'super' keyword
Lecture 5: Section7_05 – Polymorphism Concepts
Lecture 6: Section7_06 – The Virtual Method Invocation and Heterogeneous Arrays
Lecture 7: Section7_07 – Polymorphic Arguments, the 'instanceof' operator and obj cast p1
Lecture 8: Section7_08 – Polymorphic Arguments, the 'instanceof' operator and obj cast p2
Instructors
-
LearningWhilePracticing LWP
We aim to teach in a fun and useful way! -
Lawrence Decamora
Oracle Certified Java Programmer
Rating Distribution
- 1 stars: 2 votes
- 2 stars: 0 votes
- 3 stars: 1 votes
- 4 stars: 16 votes
- 5 stars: 65 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Complete Social Media Marketing Course 2024 -32 Courses in 1
- Master Course in Sales Funnel and Landing Page Optimization
- Email Marketing. Increase Sales With Email Marketing!
- Facebook Engagement Workshop: Growth Without Facebook Ads
- Unofficial Udemy Coupon Codes and Promotional Announcements
- Email marketing: Build an email list of your ideal buyers
- Mastering Business Idea Validation Online
- Social Media For Business Strategy
- Managing a waitlist in Senior Housing 101
- The Startup SPIN Method: Get Free PR with Zero Experience
- Photography Fundamentals for Beginners
- Facebook Ads & Facebook Marketing 2024 – 20 Courses in 1
- TikTok Marketing Domination : Mastering Marketing Strategies
- GetResponse Marketing Automation
- Google Business Profile SEO: Fast Google Maps Ranking
- Mailchimp Email Marketing Automation with ChatGPT
- Artificial Intelligence Powered Digital Marketing
- The complete Facebook groups Masterclass for personal brands
- Creating Amazing Videos Using Artificial Intelligence.
- Ai Youtube Masterclass: Unleash the Passive Income