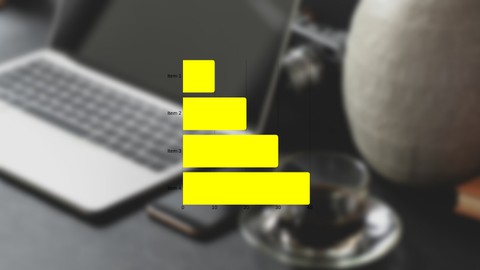
Sorting Algorithms using Java & C: Make Your Basics Strong
Sorting Algorithms using Java & C: Make Your Basics Strong, available at $69.99, has an average rating of 4.1, with 59 lectures, 9 quizzes, based on 205 reviews, and has 2356 subscribers.
You will learn about How to analyse an algorithm, understanding worst case, best case and average case complexities, Use Big O, Big Omega and Big Theta notations. Seven (7) important comparison based sorting algorithms, #Bubble Sort, #Selection Sort, #Insertion Sort, #Shell Sort, #Quick Sort, #Merge Sort and #Heap Sort. Students will get to know details of #heap data structures along with heap operations while leaning heap sort. They will experience and understand how to execute program on various input sizes and compare the execution time between different input sizes using graph. This course is ideal for individuals who are "Sorting algorithms" are gateway to the world of understanding algorithms, any programming aspirant who wants to make the fundamentals more concrete. The fact is, in Job interviews of many questions are asked from this section. It is particularly useful for "Sorting algorithms" are gateway to the world of understanding algorithms, any programming aspirant who wants to make the fundamentals more concrete. The fact is, in Job interviews of many questions are asked from this section.
Enroll now: Sorting Algorithms using Java & C: Make Your Basics Strong
Summary
Title: Sorting Algorithms using Java & C: Make Your Basics Strong
Price: $69.99
Average Rating: 4.1
Number of Lectures: 59
Number of Quizzes: 9
Number of Published Lectures: 59
Number of Published Quizzes: 9
Number of Curriculum Items: 72
Number of Published Curriculum Objects: 72
Original Price: $109.99
Quality Status: approved
Status: Live
What You Will Learn
- How to analyse an algorithm, understanding worst case, best case and average case complexities, Use Big O, Big Omega and Big Theta notations.
- Seven (7) important comparison based sorting algorithms, #Bubble Sort, #Selection Sort, #Insertion Sort, #Shell Sort, #Quick Sort, #Merge Sort and #Heap Sort.
- Students will get to know details of #heap data structures along with heap operations while leaning heap sort.
- They will experience and understand how to execute program on various input sizes and compare the execution time between different input sizes using graph.
Who Should Attend
- "Sorting algorithms" are gateway to the world of understanding algorithms, any programming aspirant who wants to make the fundamentals more concrete. The fact is, in Job interviews of many questions are asked from this section.
Target Audiences
- "Sorting algorithms" are gateway to the world of understanding algorithms, any programming aspirant who wants to make the fundamentals more concrete. The fact is, in Job interviews of many questions are asked from this section.
This course will help to understand seven most important comparison based sorting algorithms along with the details of how to estimate the complexities for any algorithm. Students will clearly understand how to estimate the best case, average case and worst case complexities for any algorithm along with details analysis of each of the sorting algorithm.
The seven sorting algorithms that you will learn in this course are as follows:
-
Bubble sort
-
Selection Sort
-
Insertion Sort
-
Shell Sort
-
Quick Sort
-
Merge Sort
-
Heap Sort
Students will learn details of heap data structures along with the heap operations like, insertion into heap, heap adjust, heap delete and heapify while learning the heap sort.
Although, sorting utilities can be found in the library of any modern day programming language, however, it is must for a programming student to understand them from scratch as this will help to form strong foundation on algorithm. Also, it is often found that, many questions are asked on sorting algorithms on Job interviews, hence, it will be really fruitful to have a strong hold on this topic.
In the course, I described the logic of each of the seven comparison based sorting algorithms using visual description that is really easy to understand, then I explained the algorithm, analysed them for their performance and finally implemented them using C and Java.
If you are interested of implementing them using in other language you can also do that following the lectures. It will be really easy to do.
Course Curriculum
Chapter 1: Introduction
Lecture 1: Introduction
Chapter 2: Efficiency of algorithm
Lecture 1: Introduction to the concept of efficiency of algorithm.
Lecture 2: Mathematical Approach for calculating efficiency
Lecture 3: How to estimate for efficiency – Big-Oh notation.
Lecture 4: Calculating Big-Oh
Lecture 5: Calculating Big-Oh continue…
Lecture 6: Calculating Big-Oh more examples
Lecture 7: Best case complexity
Lecture 8: Average case complexity
Chapter 3: Bubble Sort
Lecture 1: Bubble sort logic.
Lecture 2: Analysis of bubble sort – Worst case complexity
Lecture 3: Improve bubble sort algorithm for best case
Lecture 4: Implementation of bubble sort using C – Part 1, Understand the helper functions
Lecture 5: Implementation of bubble sort using C – Part 2 Writing the function.
Lecture 6: Implementation of bubble sort using C – Part 3 Testing
Lecture 7: Implementation of bubble sort using C – Part 4, how it behaves on sorted input.
Lecture 8: Implementation of bubble sort using Java – Part 1
Lecture 9: Implementation of bubble sort using Java – Part 2
Lecture 10: Implementation of bubble sort using Java – Part 3
Chapter 4: Selection Sort
Lecture 1: Understand Selection Sort logic and develop the algorithm
Lecture 2: Analysis of selection sort
Lecture 3: Implementation of selection sort using C
Lecture 4: Implementation of selection sort using Java
Chapter 5: Insertion Sort
Lecture 1: Introduction to insertion sort, understanding the logic using visual description
Lecture 2: Insertion sort on sorted array as input
Lecture 3: Worst case behaviour of insertion sort.
Lecture 4: Implementation of insertion sort using C
Lecture 5: Implementation of insertion sort using Java
Chapter 6: Shell Sort
Lecture 1: Introduction to Shell Sort, understand the logic with visual description.
Lecture 2: Algorithm of Shell Sort
Lecture 3: Implementation of Shell Sort using C
Lecture 4: Implementation of Shell Sort using Java
Chapter 7: Quick Sort
Lecture 1: Quick Sort introduction.
Lecture 2: Quick sort procedure with visual description.
Lecture 3: Partition logic. Understand clearly how partition logic works
Lecture 4: Dry run of Partition logic algorithm.
Lecture 5: Analysis of quick sort – average case execution time.
Lecture 6: Analysis of quick sort – worst case, when the input is sorted.
Lecture 7: Analysis of quick sort – space complexity
Lecture 8: Implementation of Quick sort using C
Lecture 9: Implementation of Quick sort using Java
Chapter 8: Merge Sort
Lecture 1: Introduction to Merge Sort
Lecture 2: Merge logic – How to merge two sorted halves of same array into one sorted array
Lecture 3: Merge sort procedure
Lecture 4: Analysis of merge sort.
Lecture 5: Implementation of merge sort using C
Lecture 6: Implementation of merge sort using Java
Chapter 9: Heap data structure and Heap sort
Lecture 1: Introduction to heap sort.
Lecture 2: Brief idea of Binary Tree
Lecture 3: Almost complete binary tree.
Lecture 4: How to representation of almost complete binary tree using 1 dimensional array.
Lecture 5: Definition of Heap.
Lecture 6: How to insert into heap.
Lecture 7: How to delete from heap.
Lecture 8: Heap adjust.
Lecture 9: Heapify – build a heap from any arbitrary array.
Lecture 10: Heap sort – understand how we can sort an array using a heap.
Lecture 11: Implementation of heap sort using C
Lecture 12: Implementation of heap sort using Java
Instructors
-
Shibaji Paul
Programming Instructor with 16+ years of experience
Rating Distribution
- 1 stars: 5 votes
- 2 stars: 4 votes
- 3 stars: 21 votes
- 4 stars: 57 votes
- 5 stars: 118 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Project Management
- Life Insurance Agent's 1-Day Lead Generation System
- How to Hire Virtual Assistants and Outsource Your Business
- Advanced Business Analysis
- B2B Mastering the Sales Process to Top Sales Agent
- Kindle Mastery 101
- Business Writing Mastery: Copywriting, Reports & More
- Crash Course: Project Management Fundamentals [Earn 6 PDUs]
- The Advanced Real Estate Financial Modeling Bootcamp
- Twitter Business Mastery – Twitter Home Business Empire
- Creating and selling digital products from scratch
- Succesful Presentations
- Sales Skills Training: Consultative Selling Master Class
- Advanced Business Writing Skills
- How to Better Understand Customer Service
- Bias effect at the work
- Winning Communication Skills for Telephone, Conference Calls
- Project Management: How to create a complete Plan in 1h
- Tableau Prep Masterclass- With real world business problems
- Alibaba Masterclass (2018)