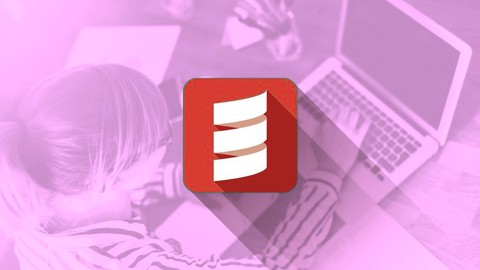
Scala Advanced, Part 3 – Functional Programming, Performance
Scala Advanced, Part 3 – Functional Programming, Performance, available at $74.99, has an average rating of 4.65, with 96 lectures, 5 quizzes, based on 109 reviews, and has 1268 subscribers.
You will learn about Advanced Functional Programming, Tail Calls, Trampolines, Functors, Monads, Applicative Functors, Different Monad Patterns Macros, Scala Compiler Phases, Abstract Syntax Trees, Quasiquotes, When and how to use macros Parser Combinators, External DSLs, Grammar Translation, Transformers, Parsing, Packrat Parsers Performance Optimization, JVM Performance Tricks, Profiling, Visual VM This course is ideal for individuals who are Scala developers looking to improve their skills, write libraries and APIs for others or Developers wanting to improve their knowledge of more advanced functional programming concepts or Developers wishing to learn how to save time and money by profiling and optimizing applications or Anyone who wants to be able to parse and use external DSLs in Scala It is particularly useful for Scala developers looking to improve their skills, write libraries and APIs for others or Developers wanting to improve their knowledge of more advanced functional programming concepts or Developers wishing to learn how to save time and money by profiling and optimizing applications or Anyone who wants to be able to parse and use external DSLs in Scala.
Enroll now: Scala Advanced, Part 3 – Functional Programming, Performance
Summary
Title: Scala Advanced, Part 3 – Functional Programming, Performance
Price: $74.99
Average Rating: 4.65
Number of Lectures: 96
Number of Quizzes: 5
Number of Published Lectures: 96
Number of Published Quizzes: 5
Number of Curriculum Items: 101
Number of Published Curriculum Objects: 101
Original Price: $19.99
Quality Status: approved
Status: Live
What You Will Learn
- Advanced Functional Programming, Tail Calls, Trampolines, Functors, Monads, Applicative Functors, Different Monad Patterns
- Macros, Scala Compiler Phases, Abstract Syntax Trees, Quasiquotes, When and how to use macros
- Parser Combinators, External DSLs, Grammar Translation, Transformers, Parsing, Packrat Parsers
- Performance Optimization, JVM Performance Tricks, Profiling, Visual VM
Who Should Attend
- Scala developers looking to improve their skills, write libraries and APIs for others
- Developers wanting to improve their knowledge of more advanced functional programming concepts
- Developers wishing to learn how to save time and money by profiling and optimizing applications
- Anyone who wants to be able to parse and use external DSLs in Scala
Target Audiences
- Scala developers looking to improve their skills, write libraries and APIs for others
- Developers wanting to improve their knowledge of more advanced functional programming concepts
- Developers wishing to learn how to save time and money by profiling and optimizing applications
- Anyone who wants to be able to parse and use external DSLs in Scala
The Escalate Software Scala Advanced course is intended for experienced Scala developers looking to improve their skills, particularly for library and API design and development. It covers topics needed to be effective in producing high quality, correct, powerful and flexible Scala libraries that are still easy to use by others.
This course assumes you have day-to-day Scala development skills equivalent to having taken the Scala Advanced course parts 1 and 2 from Escalate Software. If you have trouble understanding or following the concepts in this course because some of the concepts being presented are assuming something you are unfamiliar with, then we would recommend you check out the Applied courses as these will answer many of your questions.
Part 3 covers advanced functional programming concepts and patterns, use of Macros, how to write external DSLs with the parser-combinator library, and how to effectively optimize code by analyzing performance:
-
Functional Programming Building Blocks: ADTs, trampolines, recursion, functions
-
Functional Programming Patterns: Functors, Monads, Applicative Functors
-
Common Functional Patterns: IO, Reader, Writer, State, Free
-
Macros and Quasiquotes
-
External DSLs and Scala’s Parser-Combinator Library
-
Profiling and Optimization
-
Code Performance Considerations
It is recommended that you complete Scala Advanced parts 1 and 2 before taking this part. While not strictly necessary, we may assume knowledge from parts 1 and 2 in some of the explanations that could be hard to follow unless you know the material.
This is the final part of the Advanced Scala course. We hope you enjoyed the course and that the material proves useful.
Course Curriculum
Chapter 1: Setup Instructions
Lecture 1: Introduction
Chapter 2: Module 12 – FP part 1, Tail Calls, Trampolines, ADTs
Lecture 1: Module 12 – 01 – Introduction
Lecture 2: Module 12 – 02 – Agenda
Lecture 3: Module 12 – 03 – Recursion vs Loops
Lecture 4: Module 12 – 04 – Recursive Factorial
Lecture 5: Module 12 – 05 – Tail Recursive Factorial
Lecture 6: Module 12 – 06 – Puzzler – Fibonacci
Lecture 7: Module 12 – 07 – Mutual Calling Functions
Lecture 8: Module 12 – 08 – Recursive Even/Odd
Lecture 9: Module 12 – 09 – ADTs Recap
Lecture 10: Module 12 – 10 – First Trampoline
Lecture 11: Module 12 – 11 – Even/Odd Trampoline
Lecture 12: Module 12 – 12 – Using Trampolines
Lecture 13: Module 12 – 13 – Scala TailCalls
Lecture 14: Module 12 – 14 – Higher Order Functions Recap
Chapter 3: Module 13 – Functors, Monads, Applicative Functors
Lecture 1: Module 13 – 01 – Introduction
Lecture 2: Module 13 – 02 – Agenda
Lecture 3: Module 13 – 03 – Options Recap
Lecture 4: Module 13 – 04 – Implementing Option
Lecture 5: Module 13 – 05 – A Functor
Lecture 6: Module 13 – 06 – A Monad
Lecture 7: Module 13 – 07 – Optional In Use
Lecture 8: Module 13 – 08 – Guards, withFilter
Lecture 9: Module 13 – 09 – Testing the Guard
Lecture 10: Module 13 – 10 – Functor Laws
Lecture 11: Module 13 – 11 – Monad Laws
Lecture 12: Module 13 – 12 – Applicative Functors
Lecture 13: Module 13 – 13 – Applicative Functors with Functions
Lecture 14: Module 13 – 14 – Cartesian Syntax and Alternatives
Lecture 15: Module 13 – 15 – Functor/Monad Patterns
Lecture 16: Module 13 – 16 – IO
Lecture 17: Module 13 – 17 – Composing IO
Lecture 18: Module 13 – 18 – Reader
Lecture 19: Module 13 – 19 – Writer
Lecture 20: Module 13 – 20 – State
Lecture 21: Module 13 – 21 – Free
Lecture 22: Module 13 – 22 – Free ADT
Lecture 23: Module 13 – 23 – Free Composition
Lecture 24: Module 13 – 24 – Free – A Full Program
Lecture 25: Module 13 – 25 – Free, the Interpreter
Lecture 26: Module 13 – 26 – Running Free
Chapter 4: Module 14 – Macros
Lecture 1: Module 14 – 01 – Introduction
Lecture 2: Module 14 – 02 – Agenda
Lecture 3: Module 14 – 03 – First Rule of Macros
Lecture 4: Module 14 – 04 – Scala Compiler Phases
Lecture 5: Module 14 – 05 – Abstract Syntax Trees
Lecture 6: Module 14 – 06 – Macro Overview
Lecture 7: Module 14 – 07 – A Demo Macro
Lecture 8: Module 14 – 08 – Setting Up the Pieces
Lecture 9: Module 14 – 09 – A Macro That Does Nothing
Lecture 10: Module 14 – 10 – Macro With Generic Type Parameters
Lecture 11: Module 14 – 11 – Quasiquotes
Lecture 12: Module 14 – 12 – Doing More with Quasiquotes
Lecture 13: Module 14 – 13 – A Function Describing Macro
Lecture 14: Module 14 – 14 – Narrowing the Return Type
Lecture 15: Module 14 – 15 – Compiler Warnings and Errors
Lecture 16: Module 14 – 16 – Creating a Compiler Warning
Lecture 17: Module 14 – 17 – Macro Limitations
Lecture 18: Module 14 – 18 – Final Words
Chapter 5: Module 15 – Parser Combinators
Lecture 1: Module 15 – 01 – Introduction
Lecture 2: Module 15 – 02 – Agenda
Lecture 3: Module 15 – 03 – External DSLs
Lecture 4: Module 15 – 04 – A Basic Grammar
Lecture 5: Module 15 – 05 – Simple Parser Combinators
Lecture 6: Module 15 – 06 – Token Parsers
Lecture 7: Module 15 – 07 – Types and Transformers
Lecture 8: Module 15 – 08 – Richer Types
Lecture 9: Module 15 – 09 – StatementLine ADT
Lecture 10: Module 15 – 10 – Next and Goto
Lecture 11: Module 15 – 11 – BNF Translation
Lecture 12: Module 15 – 12 – The Print Statement
Lecture 13: Module 15 – 13 – The For Statement
Lecture 14: Module 15 – 14 – Complete Line, and Parsing
Lecture 15: Module 15 – 15 – Trying It Out
Lecture 16: Module 15 – 16 – Packrat Parsers
Chapter 6: Module 16 – Performance and Optimization
Lecture 1: Module 16 – 01 – Introduction
Lecture 2: Module 16 – 02 – Agenda
Lecture 3: Module 16 – 03 – When and Where to Optimize
Lecture 4: Module 16 – 04 – First Things First
Lecture 5: Module 16 – 05 – Collections
Lecture 6: Module 16 – 06 – Vector vs List
Lecture 7: Module 16 – 07 – List Head vs Tail Operations
Lecture 8: Module 16 – 08 – Arrays
Lecture 9: Module 16 – 09 – Register Based Arithmetic
Lecture 10: Module 16 – 10 – Timing Runs
Lecture 11: Module 16 – 11 – Bitwise Operations
Lecture 12: Module 16 – 12 – While Loops and Tail Calls
Lecture 13: Module 16 – 13 – While vs TailRec
Lecture 14: Module 16 – 14 – Library Calls
Lecture 15: Module 16 – 15 – sqrt, Trancendentals
Instructors
-
Dick Wall
Scala Developer
Rating Distribution
- 1 stars: 0 votes
- 2 stars: 0 votes
- 3 stars: 2 votes
- 4 stars: 39 votes
- 5 stars: 68 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Projeciler İçin Pratik 2 Boyutlu Autocad Eğitimi
- 나만의 메타버스(Metaverse) 로블록스 [건축과 인테리어]
- Formation complète Photoshop CC2019 à CC2023
- Photoshop 2020 Manipülasyon ve Retouch Dersleri
- Plaxis 2D İnşaat (Geoteknik) Mühendisliği Modelleme Programı
- Sıfırdan Autodesk Eagle ile Elektronik Kart Tasarımı
- Mobil UI/UX Tasarım Kursu
- 3ds Max 2019 – 08 recursos fundamentais antes de iniciar.
- Adobe İllustrator 2021 | Sıfırdan Başla Uzman Ol!
- Sweet Home 3D le cours complet
- 【Illustrator入門コース】初心者から始めるフラットデザイン作成講座
- Maya必須カメラ&ライト習得コースMaya Required Camera & Light Acquisition
- İleri Seviye Adobe Photoshop İpuçları
- İŞ AKIŞINIZI FUSİON 360'A AKTARMANIZ İÇİN 40 NEDEN
- Midjourney : L'essentiel pour sublimer vos images
- 【センス不要】絵を描けない83.7%のデザイナーが覚えるべき簡単なイラスト手法を解説!(重宝される)
- Adobe Character Animator | تعلم تحريك الشخصيات
- BAŞTAN SONA AUTOCAD 2020 EĞİTİM SETİ
- Photoshop CC: Warstwy, Maski i Tryby Mieszania
- Фотошоп 2020/2021 с нуля. Практика Photoshop.