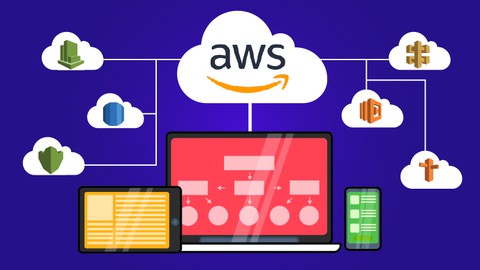
AWS Serverless REST APIs for Java Developers. CI/CD included
AWS Serverless REST APIs for Java Developers. CI/CD included, available at $89.99, has an average rating of 4.61, with 244 lectures, based on 536 reviews, and has 6333 subscribers.
You will learn about REST API and its design principles Building Serverless REST APIs in AWS with Java Amazon API Gateway Build and deploy API with AWS SAM AWS Lambda Implement User Sign-up, Sign-in and Conformation features Amazon Cognito Learn to use Cognito Authorizers Implement custom Lambda Authorizer to validate JWT Learn to Encrypt & Decrypt sensitive environment variables Learn to use API Keys and Usage Plans Unit Test AWS Lambda functions AWS CI/CD AWS CodeCommit, AWS CodeBuild, AWS CodePipeline This course is ideal for individuals who are Beginner Java developers interested in learning how to build Serverless REST APIs in Amazon Cloud It is particularly useful for Beginner Java developers interested in learning how to build Serverless REST APIs in Amazon Cloud.
Enroll now: AWS Serverless REST APIs for Java Developers. CI/CD included
Summary
Title: AWS Serverless REST APIs for Java Developers. CI/CD included
Price: $89.99
Average Rating: 4.61
Number of Lectures: 244
Number of Published Lectures: 237
Number of Curriculum Items: 244
Number of Published Curriculum Objects: 237
Original Price: $199.99
Quality Status: approved
Status: Live
What You Will Learn
- REST API and its design principles
- Building Serverless REST APIs in AWS with Java
- Amazon API Gateway
- Build and deploy API with AWS SAM
- AWS Lambda
- Implement User Sign-up, Sign-in and Conformation features
- Amazon Cognito
- Learn to use Cognito Authorizers
- Implement custom Lambda Authorizer to validate JWT
- Learn to Encrypt & Decrypt sensitive environment variables
- Learn to use API Keys and Usage Plans
- Unit Test AWS Lambda functions
- AWS CI/CD
- AWS CodeCommit, AWS CodeBuild, AWS CodePipeline
Who Should Attend
- Beginner Java developers interested in learning how to build Serverless REST APIs in Amazon Cloud
Target Audiences
- Beginner Java developers interested in learning how to build Serverless REST APIs in Amazon Cloud
AWS Serverless is probably the quickest way to build a very stable REST APIs that scale to serve millions of users. A very simple Mock API can be created and deployed in minutes. An API that uses AWS Lambda compute service will take longer but how much longer will depend on the business logic that you need to write.
In this video course, you will learn what is REST API and how to create one using AWS Serverless Services.
You will learn in detail how to use Amazon API Gateway to create REST APIs, and AWS Lambda Compute Serviceto execute business logic. The course is designed for absolute beginners, so you do not need to have any experience with AWS Serverless.
By the end of this course, you will be able to:
-
Create RESTful API endpoints,
-
Build and deploy API with AWS SAM,
-
Validate HTTP request body and request parameters,
-
Transform HTTP request body into a different model,
-
Transform HTTP response JSON into a different one,
-
Deploy APIs into different stages,
-
Perform Canary Release Deployments and shift traffic between different versions of APIs,
-
Publish multiple versions of Lambda functions and shift traffic between these functions,
-
Document and Export REST API,
-
Implement User Sign-up with Amazon Cognito,
-
Learn to use Cognito Authorizer to control who can access your API in Amazon API Gateway,
-
Implement custom Lambda Authorizer that validates JWT,
-
Secure API endpoints with API Keys and learn to configure requests Throattling and Quota,
-
Unit Test AWS Lambda functions,
-
Build CI/CD Pipeline using AWS CodeCommit, AWS CodeBuild and AWS CodePipeline developer tools.
If you want to learn how to build REST APIs quickly, without starting, configuring, and managing any servers, then this course is for you.
Course Curriculum
Chapter 1: Introduction
Lecture 1: Introduction
Lecture 2: Welcome from the instructor
Chapter 2: Introduction to REST API
Lecture 1: What is an API?
Lecture 2: Introduction to REST API
Lecture 3: REST API Resources & URIs
Lecture 4: API Operations and HTTP methods
Chapter 3: Introduction to AWS Serverless
Lecture 1: The multi-tier architecture and Microservices
Lecture 2: AWS Serverless services overview
Lecture 3: AWS Serverless architecture example
Chapter 4: AWS API Gateway
Lecture 1: Introduction
Lecture 2: Import from Swagger or Open API 3
Lecture 3: Method execution flow overview
Lecture 4: Method request overview
Lecture 5: Integration request overview
Lecture 6: Integration response overview
Lecture 7: Method response overview
Lecture 8: Trying how it works
Chapter 5: Creating Mock API Endpoints
Lecture 1: Introduction
Lecture 2: Creating a new API
Lecture 3: Creating a new Resource
Lecture 4: Creating HTTP Method
Lecture 5: Returning Mock Data
Lecture 6: Path parameter
Lecture 7: Reading the path parameter
Lecture 8: Query String Parameters
Lecture 9: Reading Query String Parameter in a Mapping template
Lecture 10: Deploying Mock API
Lecture 11: Documenting API
Chapter 6: Exporting API
Lecture 1: Introduction
Lecture 2: Export API and Test with Swagger
Lecture 3: Export API with Gateway Extensions
Lecture 4: Export API and Test with Postman
Chapter 7: Validating HTTP Request
Lecture 1: Introduction
Lecture 2: Validating Request Parameters & Headers
Lecture 3: Validating Request Body – Creating a Model.
Lecture 4: Associate Model with HTTP Request
Lecture 5: Validating Request Body – Trying how it works.
Chapter 8: Introduction to Lambda
Lecture 1: Function as a Service(SaaS)
Lecture 2: Lambda function & other Services
Lecture 3: Anatomy of a Java Lambda function handler
Lecture 4: Lambda Execution Environment
Lecture 5: Cold start, warm start & provisioned concurrency
Lecture 6: Pricing
Chapter 9: Create Java Lambda using Maven and IDE
Lecture 1: Introduction
Lecture 2: Creating new Maven project
Lecture 3: Adding Maven dependencies
Lecture 4: Maven Shade Plugin
Lecture 5: Creating Lambda function
Lecture 6: Reading Request returning Response
Lecture 7: Creating function in AWS Console
Lecture 8: Deploying Lambda function
Lecture 9: Testing Lambda function with Input Template
Lecture 10: Create new API project
Lecture 11: Assigning Lambda function to API Endpoint
Chapter 10: AWS SAM – Tools to create & deploy Lambda functions
Lecture 1: Introduction
Lecture 2: Creating a new IAM User
Lecture 3: Installing AWS SAM
Lecture 4: Installing AWS CLI
Lecture 5: Configure AWS Credentials on your computer
Lecture 6: [Updated] Creating a new project with SAM
Lecture 7: Refactor project to use a different name
Lecture 8: Read User Details JSON and return HTTP Response
Lecture 9: SAM template file overview
Lecture 10: SAM template – Resources
Lecture 11: SAM template – Outputs
Lecture 12: The event.json file
Lecture 13: Install Docker
Lecture 14: Invoke Remote Lambda function from local computer
Lecture 15: Debug Lambda function locally
Lecture 16: Deploy Lambda function to AWS
Lecture 17: Invoke public API Endpoint
Lecture 18: Viewing logs
Lecture 19: Delete AWS SAM application
Chapter 11: Data Transformations
Lecture 1: Introduction
Lecture 2: Project source code
Lecture 3: Java Map instead of APIGatewayProxy Event objects
Lecture 4: Deploying application to AWS
Lecture 5: Creating a new Request Model
Lecture 6: Creating a new Response Model
Lecture 7: Transforming HTTP Request Payload
Lecture 8: Reading New Request Model
Lecture 9: Trying how it works
Lecture 10: Response Mapping Template
Lecture 11: Configure Method Response
Lecture 12: Trying how the Response Mapping
Lecture 13: Reading with Query String Parameters & Headers
Chapter 12: Error Responses
Lecture 1: Introduction
Lecture 2: Project source code(Proxy Integration)
Instructors
-
Sergey Kargopolov
Software developer
Rating Distribution
- 1 stars: 2 votes
- 2 stars: 4 votes
- 3 stars: 35 votes
- 4 stars: 160 votes
- 5 stars: 335 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Ultimate all royalty free contents for youtubers or bloggers
- Complete WhatsApp Marketing Course
- YouTube 1M Subscribers Guide – Proven ways for YouTube Subs
- Sales Funnel Blueprint For Beginners
- Instagram Business Marketing Game Plan 10k+ Paying Customers
- Earning Online Made Easy: CPA Marketing for Beginners
- MarketHealth Mastery: Dominating Sales in 90 Days
- How to Use Instagram Video for Business and Building a Brand
- YouTube Piggyback Method – Unlimited Cheap Traffic
- Learning Search Engine Optimization (SEO) from Scratch
- Rock Your Newsletter
- Google My Business – Complete Listing Optimization Training
- Workflow in Salesforce
- Mastering Invideo AI – A Complete Guide to Text-to-Video
- Ultimate Content Marketing Workshop ⎢Certificate Course 2024
- Copywriting For Beginners Course- Start Making Money Today
- Mastering Facebook Ads: From Beginner to Expert
- Content Creation with Midjourney
- Search Engine Marketing (SEM): #1 Step-by-Step Tutorial
- The Sales Funnel Cheat Sheet Collection – 4 Courses In 1