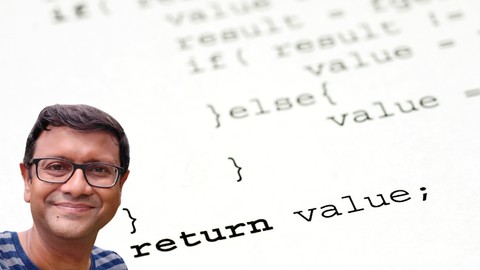
Fundamental Data Structures & Algorithms using C language.
Fundamental Data Structures & Algorithms using C language., available at $69.99, has an average rating of 4.48, with 124 lectures, 13 quizzes, based on 882 reviews, and has 7412 subscribers.
You will learn about Recursion, Stack, Polish Notations, infix postfix, FIFO, Circular & Double Ended Queue, Linked List – Linear, Double & Circular, Stack & Queue using Linked List What is stack, algorithms for Push and Pop operation. Implementation of Stack data structure using C. Using Stack – checking parenthesis in an expression Using Stack – Understanding Polish notations, algorithm and implementation of infix to postfix conversion and evaluation of postfix expression What is a FIFO Queue, understanding Queue operations – Insert and delete, implementing FIFO Queue Limitations of FIFO queue, concept of Circular Queue – Implementation of Circular queue. Concept of Double ended queue, logic development and implementation of double ended queue. Concept of Linked List – definition, why we need linked list. Singly Linked List – developing algorithms for various methods and then implementing them using C programming Doubly Linked List – developing algorithm of various methods and then implementing them using C programming Circular Linked List – developing algorithm of various methods and then implementing them using C programming How to estimate time complexity of any algorithm. Big Oh, Big Omega and Big Theta notations. Recursion, concept of Tail recursion, Recursion Vs Iteration. Binary Tree, definition, traversal (in-order, pre-order and post-order), binary search tree, implementation. Heap – concept, definition, almost complete binary tree, insertion into heap, heap adjust, deletion, heapify and heap sort. This course is ideal for individuals who are Students who want to prepare themselve for interview of top companies like Google, Amazon or Microsoft. or Students who are looking forward to be an efficient programmer, who are having data structures in their syllabus. or Students who wants to have in dept knowledge about the Stack, Queue and Linked List, Efficiency of Algorithm, Binary Tree, Heap It is particularly useful for Students who want to prepare themselve for interview of top companies like Google, Amazon or Microsoft. or Students who are looking forward to be an efficient programmer, who are having data structures in their syllabus. or Students who wants to have in dept knowledge about the Stack, Queue and Linked List, Efficiency of Algorithm, Binary Tree, Heap.
Enroll now: Fundamental Data Structures & Algorithms using C language.
Summary
Title: Fundamental Data Structures & Algorithms using C language.
Price: $69.99
Average Rating: 4.48
Number of Lectures: 124
Number of Quizzes: 13
Number of Published Lectures: 124
Number of Published Quizzes: 13
Number of Curriculum Items: 137
Number of Published Curriculum Objects: 137
Original Price: $189.99
Quality Status: approved
Status: Live
What You Will Learn
- Recursion, Stack, Polish Notations, infix postfix, FIFO, Circular & Double Ended Queue, Linked List – Linear, Double & Circular, Stack & Queue using Linked List
- What is stack, algorithms for Push and Pop operation. Implementation of Stack data structure using C.
- Using Stack – checking parenthesis in an expression
- Using Stack – Understanding Polish notations, algorithm and implementation of infix to postfix conversion and evaluation of postfix expression
- What is a FIFO Queue, understanding Queue operations – Insert and delete, implementing FIFO Queue
- Limitations of FIFO queue, concept of Circular Queue – Implementation of Circular queue.
- Concept of Double ended queue, logic development and implementation of double ended queue.
- Concept of Linked List – definition, why we need linked list.
- Singly Linked List – developing algorithms for various methods and then implementing them using C programming
- Doubly Linked List – developing algorithm of various methods and then implementing them using C programming
- Circular Linked List – developing algorithm of various methods and then implementing them using C programming
- How to estimate time complexity of any algorithm. Big Oh, Big Omega and Big Theta notations.
- Recursion, concept of Tail recursion, Recursion Vs Iteration.
- Binary Tree, definition, traversal (in-order, pre-order and post-order), binary search tree, implementation.
- Heap – concept, definition, almost complete binary tree, insertion into heap, heap adjust, deletion, heapify and heap sort.
Who Should Attend
- Students who want to prepare themselve for interview of top companies like Google, Amazon or Microsoft.
- Students who are looking forward to be an efficient programmer, who are having data structures in their syllabus.
- Students who wants to have in dept knowledge about the Stack, Queue and Linked List, Efficiency of Algorithm, Binary Tree, Heap
Target Audiences
- Students who want to prepare themselve for interview of top companies like Google, Amazon or Microsoft.
- Students who are looking forward to be an efficient programmer, who are having data structures in their syllabus.
- Students who wants to have in dept knowledge about the Stack, Queue and Linked List, Efficiency of Algorithm, Binary Tree, Heap
This course will help the students ability to grasp the knowledge of data structures and algorithm using the C programming language. Knowledge of Data Structures and Algorithms are essential in developing better programming skills.
This course is based on the standard curriculum of Universities across the globe for graduate level engineering and computer application course.
Apart from step by step development of concepts students will also learn how to write algorithms and then how to write programs based on the algorithms in this course.
You will learn the following in this course: (All implemented using C programming)
-
Fundamental of Data Structure concept
-
Why we need Data Structures
-
Stack – Idea, definition, algorithm, implementations.
-
Using Stack – Parenthesis checking, Polish Notation, Infix to postfix conversion and evaluation.
-
FIFO Queue – Idea, definition, algorithm, implementation.
-
Circular Queue using array – Idea, definition, algorithm, implementation.
-
Double ended queue using array – Idea, definition, algorithm, implementation.
-
Linked List – Idea, definition, why we need linked list. Comparison with array.
-
Singly Linked List – Development of algorithm for various operations and then Implementation of each of them
-
Creating Stack and Queue using Singly Linked list – Implementation.
-
Doubly Linked List – Idea, definition, algorithm of various operations and implementations.
-
Circular Linked List – Idea, definition, algorithm and implementations.
14. Calculating efficiency of algorithms, Worst Case (Big Oh), Average Case (Big Theta) and Best case (Big omega) complexities. How to calculate them for different algorithms.
15. Binary Searching
16. Recursion in detail. Example program using recursion and the critical comparison between Recursive approach and Iterative approach of problem solving.
17. Binary Tree, definition, traversal (In, Pre and Post Order), Binary Search Tree implementation.
18. Heap data structure, definition, heap insertion, deletion, heap adjust, Heapify and heap sort.
Course Curriculum
Chapter 1: Introduction to the course.
Lecture 1: Introduction to the course.
Chapter 2: All about Stack
Lecture 1: Introduction of Stack
Lecture 2: Some practical example where Stack is used.
Lecture 3: Basic Algorithm for Stack data structure.
Lecture 4: Implementation of Stack.
Lecture 5: Some more explanations about the use of Pointers
Lecture 6: Building a menu for the implementation.
Lecture 7: Make the Stack dynamic.
Lecture 8: Make the stack more dynamic.
Lecture 9: Stack In Action – Decimal to binary conversion
Lecture 10: Stack In Action – Reversing the content of a text file.
Chapter 3: Step-by-step developing a parenthesis checking program using Stack.
Lecture 1: Understanding the problem.
Lecture 2: Developing the algorithm for bracket checking.
Lecture 3: The explanation of the algorithm that we develop for parenthesis checking.
Lecture 4: Implementation of parenthesis checking program – Part 1
Lecture 5: Implementation of parenthesis checking program – Part 2
Chapter 4: Polish notation and Reverse Polish Notation.
Lecture 1: Introduction to Polish Notation
Lecture 2: Understanding precedence of operators, conversion idea – infix to prefix/postfix
Lecture 3: How to evaluate Polish or Reverse Polish Notations.
Lecture 4: Algorithm for evaluating Postfix expression.
Lecture 5: Implementing evaluation of Postfix expression with C Programming language.
Lecture 6: Discussion on how to convert Infix to Postfix.
Lecture 7: Infix to Postfix conversion – More examples with procedure
Lecture 8: Elaboration of the procedure that converts infix to postfix.
Lecture 9: Writing the algorithm for converting Infix expression to equivalent Postfix.
Lecture 10: Dry running the Algorithm for converting Infix to Postfix.
Lecture 11: Starting the implementation, lets first develop the precedence checker function.
Lecture 12: Writing the C function for converting Infix to Postfix.
Lecture 13: Combine the conversion and evaluation function in a single program.
Chapter 5: All about Queue
Lecture 1: Introduction to Queue
Lecture 2: The FIFO queue implementation idea using Array – Understanding with animation.
Lecture 3: Algorithm for FIFO Queue.
Lecture 4: Dry run the FIFO queue algorithm.
Lecture 5: Implementation of FIFO Queue.
Lecture 6: A menu for the Queue program.
Lecture 7: The loophole in our implementation of FIFO Queue.
Lecture 8: Understanding the loophole, why that happened?
Lecture 9: Introduction to Circular Queue.
Lecture 10: Circular queue operations. How to perform enqueue and dequeue operations.
Lecture 11: Algorithm for Circular Queue operations.
Lecture 12: Implementation of Circular Queue.
Lecture 13: Introduction to Double Ended Queue
Lecture 14: Algorithm development for Double Ended Queue operations.
Lecture 15: Dry run of the DEQ algorithm.
Lecture 16: Implementation of Double Ended Queue.
Chapter 6: Linked List
Lecture 1: Introduction to Linked List.
Lecture 2: Definition of Linked List, conception of Node, understanding basic terminologies
Lecture 3: Categories of Linked List – Singly, Doubly and Circular Linked List.
Chapter 7: Singly Linked List
Lecture 1: Understanding the 'struct' type we need for implementing singly linked list.
Lecture 2: The Singly Linked List operations – starting the program.
Lecture 3: Developing Insert At Tail operation – Add a new node as last node.
Lecture 4: Implementing Insert at Head – Add a new node as the first node.
Lecture 5: Traversing the linked list – printing the content of each node.
Lecture 6: Printing the detail of each node of the linked list.
Lecture 7: Compiling and executing the program written so far.
Lecture 8: Practice for Singly Linked List
Lecture 9: Developing find operation – to search for a target in the linked list.
Lecture 10: Load data from file and build the linked list.
Lecture 11: Creating Linked List from randomly generated integer numbers.
Lecture 12: Delete first operation to delete the first node.
Lecture 13: Delete last operation to delete the last node.
Lecture 14: Delete a node that contain a target data.
Lecture 15: Reverse the linked list.
Lecture 16: Traverse the singly linked list recursively.
Lecture 17: Implementation of Stack using singly linked list.
Lecture 18: Implementation of Queue using Linked List
Chapter 8: Doubly Linked List
Lecture 1: Introduction to Doubly Linked List.
Lecture 2: Starting the program to implement various operations for Doubly Linked List.
Lecture 3: Implementation of Add First method to add a new node as the first node.
Lecture 4: AddLast implementation to add a new node as the last node.
Lecture 5: Find and Insert After and Insert Before operation.
Lecture 6: Deleting a node – delete first, delete last and delete a target.
Lecture 7: Double Ended Queue using doubly linked list.
Chapter 9: Circular Linked List.
Lecture 1: Introduction to Circular Linked List.
Lecture 2: Insert operation for Circular Linked List.
Lecture 3: Delete Node operation.
Lecture 4: Developing find and print operation.
Chapter 10: Efficiency of Algorithm
Instructors
-
Shibaji Paul
Programming Instructor with 16+ years of experience
Rating Distribution
- 1 stars: 6 votes
- 2 stars: 16 votes
- 3 stars: 97 votes
- 4 stars: 317 votes
- 5 stars: 446 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- The Millionaire Marketer: A Blueprint for Digital Wealth
- Adobe Spark : The Complete Guide To Adobe Spark
- Instagram Marketing Business Coach Certification Coaching
- Unbounce for Beginners: Build a Landing Page with Unbounce
- How to Use Facebook Ads to Find Lots Of Paying Customers
- YouTube Newbies: Start your Channel Fast, Easy and Simple
- Author School Visits 101: Children's Book Marketing
- The Ultimate Instagram Growth Hacking Course
- Video Marketing Masterclass
- Psychology for Managers & Entrepreneurs
- Marketing 101 for Self-Published Authors
- Pinterest Marketing from A to Z for Beginners
- Complete Google Adwords For Video: Boost Your YouTube Views!
- Launch-make your business profitable and easy
- Reddit Marketing For Business
- Retargeting and Facebook Ads Retargeting made Easy & Simple
- Affiliate Success Blueprint: ChatGPT, Canva & Pinterest
- Mastering Account-Based Marketing (ABM)
- AI-Powered Email Marketing: A Complete Guide
- PR Social Media Monitoring & Dashboards for Facebook etc