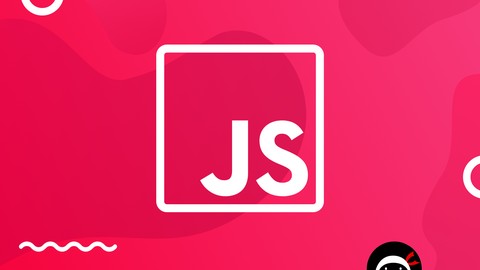
Modern JavaScript (Complete guide, from Novice to Ninja)
Modern JavaScript (Complete guide, from Novice to Ninja), available at $99.99, has an average rating of 4.77, with 188 lectures, based on 10906 reviews, and has 35816 subscribers.
You will learn about Learn how to program with modern JavaScript, from the very beginning to more advanced topics Learn all about OOP (object-oriented programming) with JavaScript, working with prototypes & classes Learn how to create real-world front-end applications with JavaScript (quizes, weather apps, chat rooms etc) Learn how to make useful JavaScript driven UI components like popups, drop-downs, tabs, tool-tips & more. Learn how to use modern, cutting-edge JavaScript features today by using a modern workflow (Babel & Webpack) Learn how to use real-time databases to store, retrieve and update application data Explore API's to make the most of third-party data (such as weather information) This course is ideal for individuals who are New or junior developers who want to learn JavaScript from the ground up or Front-end developers who want to level-up their JavaScript knowledge using modern, cutting-edge techniques or Back-end developers who want to learn the JavaScript language for front-end development It is particularly useful for New or junior developers who want to learn JavaScript from the ground up or Front-end developers who want to level-up their JavaScript knowledge using modern, cutting-edge techniques or Back-end developers who want to learn the JavaScript language for front-end development.
Enroll now: Modern JavaScript (Complete guide, from Novice to Ninja)
Summary
Title: Modern JavaScript (Complete guide, from Novice to Ninja)
Price: $99.99
Average Rating: 4.77
Number of Lectures: 188
Number of Published Lectures: 188
Number of Curriculum Items: 188
Number of Published Curriculum Objects: 188
Original Price: £99.99
Quality Status: approved
Status: Live
What You Will Learn
- Learn how to program with modern JavaScript, from the very beginning to more advanced topics
- Learn all about OOP (object-oriented programming) with JavaScript, working with prototypes & classes
- Learn how to create real-world front-end applications with JavaScript (quizes, weather apps, chat rooms etc)
- Learn how to make useful JavaScript driven UI components like popups, drop-downs, tabs, tool-tips & more.
- Learn how to use modern, cutting-edge JavaScript features today by using a modern workflow (Babel & Webpack)
- Learn how to use real-time databases to store, retrieve and update application data
- Explore API's to make the most of third-party data (such as weather information)
Who Should Attend
- New or junior developers who want to learn JavaScript from the ground up
- Front-end developers who want to level-up their JavaScript knowledge using modern, cutting-edge techniques
- Back-end developers who want to learn the JavaScript language for front-end development
Target Audiences
- New or junior developers who want to learn JavaScript from the ground up
- Front-end developers who want to level-up their JavaScript knowledge using modern, cutting-edge techniques
- Back-end developers who want to learn the JavaScript language for front-end development
Hey gang, and welcome to your first step on the path to becoming a JavaScript ninja! In this course I’ll be teaching you my absolute favourite language (JavaScript!) from the very beginning, right through to creating fully-fledged, dynamic & interactive web experiences.
We’ll cover all the basics to get you up-and-running quickly, before diving in to some of the really fun stuff like web-page manipulation, creating interactive forms, popups & other cool effects. Along the way we’ll be using the latest additions to the JavaScript specification (ES6, 7 & beyond) and maintaining good coding standards to keep our code clean and effective!
Once we master the basics, we’ll dive into several real-life JavaScript projects, including an interactive quiz, a weather app, a real-time chat application and a small UI library you can use in all your future projects!
We’ll also take a look at some more advanced topics – object oriented programming, asynchronous code, real-time databases using Firebase (including a new chapter about Firebase 9) and much more. Finally, we’ll be setting up a modern work-flow using Webpack & Babel, so that by the end of this course you’ll be no less than a black-belt JavaScript developer with a lot of coding techniques in your tool-belt.
Speaking of ninjas, I’m also known as The Net Ninja on YouTube, where you’ll find hundreds of free coding tutorials, so feel free to pop by to say hello :).
Course Curriculum
Chapter 1: Introduction
Lecture 1: Why You Should Take This Course
Lecture 2: Why JavaScript is Amazing
Lecture 3: Setting up Your Environment
Lecture 4: Course Files
Chapter 2: JavaScript Basics
Lecture 1: Adding JavaScript to a Web Page
Lecture 2: The Browser Console
Lecture 3: Variables, Constants & Comments
Lecture 4: Data Types at a Glance
Lecture 5: Strings
Lecture 6: Common String Methods
Lecture 7: Numbers
Lecture 8: Template Strings
Lecture 9: Arrays
Lecture 10: Null & Undefined
Lecture 11: Booleans & Comparisons
Lecture 12: Loose vs Strict Comparison
Lecture 13: Type Conversion
Chapter 3: Control Flow Basics
Lecture 1: What is Control Flow?
Lecture 2: For Loops
Lecture 3: While Loops
Lecture 4: Do While Loops
Lecture 5: If Statements
Lecture 6: Else & Else If
Lecture 7: Logical Operators
Lecture 8: Logical NOT
Lecture 9: Break & Continue
Lecture 10: Switch Statements
Lecture 11: Variables & Block Scope
Chapter 4: Functions & Methods
Lecture 1: What are Functions?
Lecture 2: Function Declarations & Expressions
Lecture 3: Arguments & Parameters
Lecture 4: Returning Values
Lecture 5: Arrow Functions
Lecture 6: Functions vs Methods
Lecture 7: Foreach Method & Callbacks
Lecture 8: Callback Functions in Action
Chapter 5: Object Literals
Lecture 1: Objects at a Glance
Lecture 2: Creating an Object Literal
Lecture 3: Adding Methods
Lecture 4: 'this' Keyword
Lecture 5: Objects in Arrays
Lecture 6: Math Object
Lecture 7: Primitive vs Reference Types
Chapter 6: The Document Object Model
Lecture 1: Interacting with the Browser
Lecture 2: The DOM Explained
Lecture 3: The Query Selector
Lecture 4: Other Ways to Query the DOM
Lecture 5: Adding & Changing Page Content
Lecture 6: Getting & Setting Attributes
Lecture 7: Changing CSS Styles
Lecture 8: Adding & Removing Classes
Lecture 9: Parents, Children & Siblings
Lecture 10: Event Basics (click events)
Lecture 11: Creating & Removing Elements
Lecture 12: Event Bubbling (and delegation)
Lecture 13: More DOM Events
Lecture 14: Building a Popup
Chapter 7: Forms & Form Events
Lecture 1: Events Inside Forms
Lecture 2: Submit Events
Lecture 3: Regular Expressions
Lecture 4: Testing RegEx Patterns
Lecture 5: Basic Form Validation
Lecture 6: Keyboard Events
Chapter 8: Project – Interactive Ninja Quiz
Lecture 1: Project Preview & Setup
Lecture 2: Bootstrap Basics
Lecture 3: HTML Template
Lecture 4: Checking Answers
Lecture 5: Showing the Score
Lecture 6: The Window Object
Lecture 7: Intervals & Animating the Score
Chapter 9: Array Methods
Lecture 1: Filter Method
Lecture 2: Map Method
Lecture 3: Reduce Method
Lecture 4: Find Method
Lecture 5: Sort Method
Lecture 6: Chaining Array Methods
Chapter 10: Project – Todo List
Lecture 1: Project Preview and Setup
Lecture 2: HTML & CSS Template
Lecture 3: Adding Todos
Lecture 4: Deleting Todos
Lecture 5: Searching & Filtering Todos
Chapter 11: Dates & Times
Lecture 1: Dates & Times in JavaScript
Lecture 2: Timestamps & Comparisons
Lecture 3: Building a Digital Clock
Lecture 4: Date-fns Library
Chapter 12: Async JavaScript
Lecture 1: What is Asynchronous JavaScript?
Lecture 2: Async Code in Action
Lecture 3: What are HTTP Requests?
Instructors
-
The Net Ninja (Shaun Pelling)
Online Coding Tutor & Net Ninja
Rating Distribution
- 1 stars: 33 votes
- 2 stars: 48 votes
- 3 stars: 304 votes
- 4 stars: 2377 votes
- 5 stars: 8145 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple