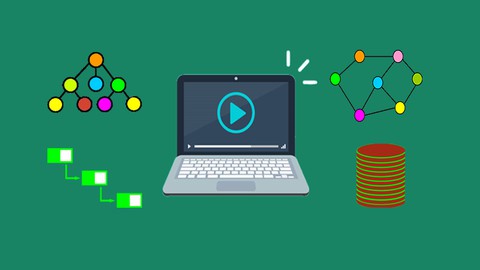
The Complete Data Structures and Algorithms Course in Java
The Complete Data Structures and Algorithms Course in Java, available at $59.99, has an average rating of 4.6, with 185 lectures, based on 467 reviews, and has 60381 subscribers.
You will learn about Fundamentals of Data Structures and Algorithms Become Confident in Data Structures and Algorithms Learn, Implement and Use Different Data Structure Learn Popular Data Structures and Their Algorithms Master Algorithms including 7 Sorting Algorithms Become Confident in Algorithms Coding Interview This course is ideal for individuals who are Any one who wants to build strong foundation on data structures and algorithms or Anyone who wants to work at FAANG(Facebook, Apple, Amazon, Netflix, Google) Company or Any engineer, developer, programmer, who wants to improve their coding skills or Any self taught programmer who missed out on a computer science degree It is particularly useful for Any one who wants to build strong foundation on data structures and algorithms or Anyone who wants to work at FAANG(Facebook, Apple, Amazon, Netflix, Google) Company or Any engineer, developer, programmer, who wants to improve their coding skills or Any self taught programmer who missed out on a computer science degree.
Enroll now: The Complete Data Structures and Algorithms Course in Java
Summary
Title: The Complete Data Structures and Algorithms Course in Java
Price: $59.99
Average Rating: 4.6
Number of Lectures: 185
Number of Published Lectures: 179
Number of Curriculum Items: 185
Number of Published Curriculum Objects: 179
Original Price: $199.99
Quality Status: approved
Status: Live
What You Will Learn
- Fundamentals of Data Structures and Algorithms
- Become Confident in Data Structures and Algorithms
- Learn, Implement and Use Different Data Structure
- Learn Popular Data Structures and Their Algorithms
- Master Algorithms including 7 Sorting Algorithms
- Become Confident in Algorithms Coding Interview
Who Should Attend
- Any one who wants to build strong foundation on data structures and algorithms
- Anyone who wants to work at FAANG(Facebook, Apple, Amazon, Netflix, Google) Company
- Any engineer, developer, programmer, who wants to improve their coding skills
- Any self taught programmer who missed out on a computer science degree
Target Audiences
- Any one who wants to build strong foundation on data structures and algorithms
- Anyone who wants to work at FAANG(Facebook, Apple, Amazon, Netflix, Google) Company
- Any engineer, developer, programmer, who wants to improve their coding skills
- Any self taught programmer who missed out on a computer science degree
Welcome to “Data Structures and Algorithms for Coding Interview” course.
Want to land a job at a great tech industry like Google, Microsoft, Facebook, Netflix, Amazon, or other industries but you are intimidated by the foundation of data structures and algorithms skills for the job?
Many programmers who are “self taught”, feel that one of the main disadvantages they face compared to college educated graduates in computer science is the fact that they don’t have knowledge about algorithms, data structures and the notorious Big-O Notation. Get on the same level as someone with computer science degree by learning the fundamental building blocks of computer science which will give you a big boost during interviews.
Here is what you will learn in this course:
>> Algorithm Run Time Analysis – Big O – O(n) Notation
>> Array (1D, 2D Array)
>> Linked List (All Types of Linked List)
>> StackData Structure
>> Queue Data Structure
>> Binary Tree (Array, Linked List Implementation, BFS/DFS Traversal and more)
>> Binary Search Tree
>> Binary Heap
>> AVL Tree
>> Trie Data Structure
>> Searching Algorithms
>> Recursion
>> Sorting Algorithms
>> Dynamic Programming
>> Hashing
>> Graph*
Unlike most instructors, I am not a marketer or a salesperson. I am a self taught programmer(I studied statistics not computer science) who has worked and managed teams of engineers and have been in these interviews both as an interviewee as well as the interviewer.
Taking his experience in educational statistics and coding, Barik’s courses will take you on an understanding of complex subjects that you never thought.
We have 30 days money back guarantee, so nothing to lose here.
See you inside the courses!
Course Curriculum
Chapter 1: Introduction
Lecture 1: Introduction
Chapter 2: Algorithm Run Time Analysis (Big O Notation)
Lecture 1: What is Algorithm Runtime Analysis
Lecture 2: Why should we learn algorithm run time analysis?
Lecture 3: Time Complexity Analysis Example #1
Lecture 4: Time Complexity Analysis Example #2
Chapter 3: Data Structure – Array
Lecture 1: What is an array & why we need an array
Lecture 2: Types of array
Lecture 3: How is an array represented in RAM
Lecture 4: Create a 1D Array
Lecture 5: Traverse 1D Array
Lecture 6: Get, Insert, Update & Delete Operations in 1D Array
Lecture 7: Searching Algorithms (Linear Search + Binary Search)
Lecture 8: Create a 2D Array
Lecture 9: Get, Insert & Update Operations in 2D Array
Lecture 10: Traverse 2D Array
Lecture 11: 1D Array Problem: Move Zeroes
Lecture 12: 1D Array Problem: Remove Duplicates from Sorted Array
Lecture 13: 2D Array Problem: Rotate Image
Lecture 14: 2D Array Problem: Spiral Matrix
Chapter 4: Data Structure – Linked List
Lecture 1: What is Linked List
Lecture 2: Different Types of Linked Lists
Lecture 3: Creation & Insertion Operations in SLL (Singly Linked List)
Lecture 4: Insertion (At Index, At Begining & At End) Operations in SSL(Singly LInked List)
Lecture 5: Travering & Searching in SLL (Singly Linked List)
Lecture 6: Deleting Node & Deleting Entire Linked List (Singly Linked List)
Lecture 7: Source Code for Singly Linked List (SLL)
Lecture 8: Creation & Insertion Operations in CSLL (Circular Singly Linked List)
Lecture 9: Traversing & Searching in CSLL (Circular Singly Linked List)
Lecture 10: Insertion in CSLL (Circular Singly Linked List)
Lecture 11: Deleting Node & Deleting Entire Linked List (Circular Singly Linked List)
Lecture 12: Source Code for Circular Singly Linked List (CSLL)
Lecture 13: Creation & Insertion Operations in DLL (Doubly Linked List)
Lecture 14: Traversing & Searching in DLL (Doubly Linked List)
Lecture 15: Insertion in DLL (Doubly LInked List)
Lecture 16: Deleting Node & Deleting Enire Linked List (Doubly Linked List)
Lecture 17: Source Code for Doubly Linked List (DLL)
Lecture 18: Creation & Insertion Operations in CDLL (Circular Doubly Linked LIst)
Lecture 19: Traversing & Searching in CDLL (Circular Doubly Linked List)
Lecture 20: Insertion in CDLL (Circular Doubly Linked List)
Lecture 21: Deleting Node & Deleting Entire Linked List (Circular Doubly Linked List)
Lecture 22: Source Code for Circular Doubly Linked List (CDLL)
Lecture 23: Linked List Problem: Reverse a Singly Linked List — Recursive Solution
Lecture 24: Linked List Problem: Reverse a Singly Linked List — Iterative Solution
Chapter 5: Data Structure – Stack
Lecture 1: What is Stack
Lecture 2: Stack Implementation Options
Lecture 3: Array – Create, Push & Pop
Lecture 4: Array – Peek, isEmpty, isFull & deleteStack
Lecture 5: Source Code — Stack Using Array
Lecture 6: Linked List – Create, Push & Pop
Lecture 7: Linked List – Peek, isEmpty, & deleteStack
Lecture 8: Source Code — Stack Using Linked List
Lecture 9: Stack Problem: Valid Parentheses
Lecture 10: Stack Problem: Decode String
Chapter 6: Data Structure – Queue
Lecture 1: What is Queue
Lecture 2: Queue Implementation Options
Lecture 3: Array – Linear Queue
Lecture 4: Source Code – Linear Queue Implementation using Array
Lecture 5: Array – Circular Queue
Lecture 6: Source Code – Circular Queue
Lecture 7: Linked List – Linear Queue
Lecture 8: Source Code – Linear Queue Implementation using LInked List
Chapter 7: Data Structure – Binary Tree
Lecture 1: What is Tree & Why should we learn tree
Lecture 2: Tree Terminologies :: Root, Leaf, Edge, Ancestor, … Depth of Tree ::
Lecture 3: Tree Terminologies :: Predecessor & Successor ::
Lecture 4: What is Binary Tree
Lecture 5: Types of Binary Tree
Lecture 6: How is Tree Represented :: Linked List & Array Representation ::
Lecture 7: Create Binary Tree & Insert Value :: Linked List Implementation ::
Lecture 8: Search for a Value in Binary Tree
Lecture 9: Delete Value from Binary Tree
Lecture 10: Delete Binary Tree
Lecture 11: Tree Traversal Techniques :: Inorder, Preorder & Postorder Traversal ::
Lecture 12: Preorder Traversal Details :: Recursive + Iterative ::
Lecture 13: Inorder Traversal Details :: Recursive + Iterative ::
Lecture 14: Postorder Traversal Details :: Recursive + Iterative ::
Lecture 15: Level Order Traversal Details :: Recursive + Iterative ::
Lecture 16: Source Code :: Implementation of Binary Tree Using Linked List ::
Lecture 17: Create Binary Tree & Insert Value :: Array Implementation ::
Lecture 18: Search for a Value in Binary Tree
Lecture 19: Delete Node from Binary Tree
Lecture 20: Delete Binary Tree
Lecture 21: Preorder Traversal
Lecture 22: Inorder Traversal
Lecture 23: Postorder Traversal
Lecture 24: Level order Traversal
Lecture 25: Source Code :: Implementation of Binary Tree Using Array ::
Chapter 8: Data Structure – Binary Search Tree (BST)
Lecture 1: What & Why is BST
Lecture 2: Creation & Insertion in BST
Lecture 3: Traversing BST
Lecture 4: Searching in BST
Lecture 5: Deletion Node in BST
Lecture 6: Deletion of BST
Instructors
-
Md. A. Barik
Software Engineer | Udemy Instructor
Rating Distribution
- 1 stars: 11 votes
- 2 stars: 13 votes
- 3 stars: 72 votes
- 4 stars: 155 votes
- 5 stars: 217 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- CIPS: Procurement and Supply Environments [L3M1] – Exam Test
- Marketing on Twitter
- Blog Your Book in 30 Days – Become a Published Author
- Facebook Marketing: Power Editor Beginner's Guide
- YouTube Marketing: Mastering YouTube For Business Growth
- AI Content Marketing Fundamentals
- Google My Business SEO with AI: Google Maps Course 2024
- YouTube Channel SEO: Marketing Secrets for All Levels
- Competitive analysis: Tools to beat your competitors
- Boost Your Business With Email Marketing Secrets
- Business Growth Strategy: Marketing Plan & Strategy to Grow!
- Master Class in Managing Distribution Channels in Sales
- Introduction to Marketing
- The Complete YouTube Course 2021: Become Famous on YouTube!
- 10 Perfect Lead Magnet Ideas For Coaches And Consultants
- How to Create a Video to Sell Your Product or Service
- The Basics of Live Streaming
- Effective Listening Skills to Become More Successful
- Lean Leadership Skills, Lean Culture & Lean Management
- Upwork Freelancing Guide 2021-My Success Story