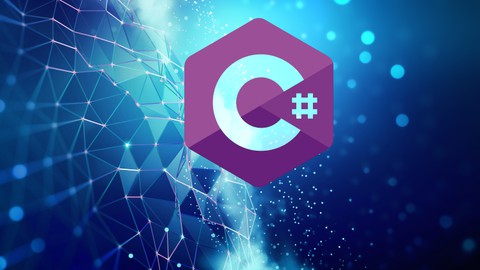
Beginning Object-oriented Programming with C#
Beginning Object-oriented Programming with C#, available at $69.99, has an average rating of 4.63, with 91 lectures, based on 980 reviews, and has 4018 subscribers.
You will learn about Understand why C# is organized the way it is Learn how to step from procedural to proper object-oriented design Construct larger classes out of smaller ones Combine behavior to construct complex features This course is ideal for individuals who are Beginner C# developers curious about modern programming practices or C# programmers who wish to improve their fundamental skills It is particularly useful for Beginner C# developers curious about modern programming practices or C# programmers who wish to improve their fundamental skills.
Enroll now: Beginning Object-oriented Programming with C#
Summary
Title: Beginning Object-oriented Programming with C#
Price: $69.99
Average Rating: 4.63
Number of Lectures: 91
Number of Published Lectures: 91
Number of Curriculum Items: 91
Number of Published Curriculum Objects: 91
Original Price: $109.99
Quality Status: approved
Status: Live
What You Will Learn
- Understand why C# is organized the way it is
- Learn how to step from procedural to proper object-oriented design
- Construct larger classes out of smaller ones
- Combine behavior to construct complex features
Who Should Attend
- Beginner C# developers curious about modern programming practices
- C# programmers who wish to improve their fundamental skills
Target Audiences
- Beginner C# developers curious about modern programming practices
- C# programmers who wish to improve their fundamental skills
In this course, you will learn the basic principles of object-oriented programming, and then learn how to apply those principles to construct an operational and correct code using the C# programming language and .NET. As the course progresses, you will learn such programming concepts as objects, method resolution, polymorphism, object composition, class inheritance, object substitution, etc., but also the basic principles of object-oriented design and even project management, such as abstraction, dependency injection, open-closed principle, tell don’t ask principle, the principles of agile software development and many more.
After completing this course, you will be qualified to continue learning the principles of object-oriented design, and to start developing applications that will be modeling different business domains.
This course differs from other similar courses in that it first devises the C# programming language from scratch, and the .NET Runtime that makes the object-oriented code run. Only after completing this task shall we step on to using the programming concepts to implement customer’s requirements in C#.
The reason for such an extreme experiment is to teach the programmers that any object-oriented language is only a tool, which relies on a small set of operations that are already implemented for us – such as silently passing the this reference or resolving function addresses from the object reference at run time. I find great danger in writing code without understanding what happens when a piece of object-oriented code is taken for execution.
Course Curriculum
Chapter 1: The Dawn of C#
Lecture 1: Introducing the C# Programming Language
Lecture 2: What Follows in This Course
Lecture 3: Installing the Visual Studio
Lecture 4: The Dawn of Computer Programming
Lecture 5: Inventing Procedural Programming Constructs
Lecture 6: Summary
Chapter 2: Inventing Objects
Lecture 1: Hitting the Limitation of Procedural Programming
Lecture 2: Seeing the First Glimpse of Objects
Lecture 3: The Dawn of Objects
Lecture 4: Speaking the Language of Objects
Lecture 5: Managing Memory Allocations
Lecture 6: Summary
Chapter 3: Introducing C# Language Syntax
Lecture 1: Introducing C# Language Syntax
Lecture 2: Outlining Code Blocks and Instructions
Lecture 3: Defining Block Instructions
Lecture 4: Method Definitions in C#
Lecture 5: Understanding Access Modifiers
Lecture 6: Understanding Program Entry Point
Lecture 7: Running the Console Application
Lecture 8: Exercise
Lecture 9: Summary
Chapter 4: Inventing Object-oriented Programming
Lecture 1: Understanding the Need to Vary Implementation
Lecture 2: Inventing Polymorphic Classes
Lecture 3: Inventing Virtual Functions
Lecture 4: Implementing Polymorphic Method Calls
Lecture 5: Understanding Virtual Functions
Lecture 6: Virtual Methods in C#
Lecture 7: Exercise
Lecture 8: Summary
Chapter 5: Introducing Visual Studio Projects and Solutions
Lecture 1: Understanding .NET Assemblies
Lecture 2: Understanding Solution and Project Elements
Lecture 3: Introducing Customer’s Requirements
Lecture 4: Designing Classes
Lecture 5: Initializing Objects
Lecture 6: Summary
Chapter 6: Designing an Object Model
Lecture 1: Adding Behavior to Classes
Lecture 2: Implementing the ToString Method
Lecture 3: Modeling the Domain with Classes
Lecture 4: Removing Code Duplication
Lecture 5: Naming Domain-related Methods
Lecture 6: Implementing Domain-related Methods
Lecture 7: Stepping from Procedural to Object-oriented Programming
Lecture 8: Providing Placeholders for Unimplemented Methods
Lecture 9: Autonomous Exercise
Lecture 10: Summary
Chapter 7: Applying the "Tell, Don't Ask" Principle to Objects
Lecture 1: Chaining Method Calls
Lecture 2: Introducing Expression-bodied Methods
Lecture 3: Introducing the “Tell, Don’t Ask” Principle
Lecture 4: Implementing Private Methods on a Class
Lecture 5: Pattern Matching Expressions in C#
Lecture 6: Completing the Calendar Model
Lecture 7: Understanding the Top-down Development Style
Lecture 8: Completing the Model
Lecture 9: Demonstrating the Model
Lecture 10: Summary
Chapter 8: Improving on Fundamental Principles of Object-oriented Design
Lecture 1: Benefiting from the Deep Domain Model
Lecture 2: Combining Existing Features to Build New Ones
Lecture 3: Understanding Method Overloading
Lecture 4: Introducing Requests for Polymorphism
Lecture 5: Ordering User Stories before Implementing Them
Lecture 6: Introducing the Open-closed Principle
Lecture 7: Isolating Varying Operations in Classes
Lecture 8: Autonomous Exercise
Lecture 9: Summary
Chapter 9: Implementing Polymorphic Classes
Lecture 1: Revisiting Dynamic Method Calls
Lecture 2: Understanding Dependencies and Dependency Injection
Lecture 3: Implementing Dependency Injection
Lecture 4: Using the Dependency
Lecture 5: Pulling Out a Derived Class
Lecture 6: Understanding Abstract Methods and Classes
Lecture 7: Practicing Object Substitution Principle
Lecture 8: Substituting Objects at Run Time
Lecture 9: Summary
Chapter 10: Reiterating Principles of Object-oriented Design
Lecture 1: How to Learn Programming
Lecture 2: Reiterating Classes and Objects
Lecture 3: Reiterating Object-oriented Design
Lecture 4: Reiterating Method Composition
Lecture 5: Reiterating Dependency Injection and Object Composition
Lecture 6: Designing for Evolution
Lecture 7: Refactoring and Redesigning Code
Lecture 8: Summary
Chapter 11: Introducing Collections and Loops
Lecture 1: Understanding the Need for Collections
Lecture 2: Looping Through the Array
Lecture 3: Initializing Arrays
Lecture 4: Understanding Collection Polymorphism
Lecture 5: Introducing Lists
Lecture 6: Introducing the While Loop
Lecture 7: Introducing Other Kinds of Loops
Lecture 8: Introducing Numeric Types
Instructors
-
Zoran Horvat
CEO and Principal Consultant at Coding Helmet s.p.r.l.
Rating Distribution
- 1 stars: 4 votes
- 2 stars: 13 votes
- 3 stars: 91 votes
- 4 stars: 331 votes
- 5 stars: 542 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Velo for Beginners: Become a Frontend Wix Code Developer
- Vue & Vuex | Vue Js Front End Web Development with Vuex
- Learn to create a 2D Racing car game for FREE PART 7.
- Beginning Object-oriented Programming with C#
- Learn how to make websites from scratch to live
- React.JS Crash Course: The Complete Course for Beginners
- 230+ Exercises – Python for Data Science – NumPy + Pandas
- Build Instagram to Master SwiftUI and Firestore
- ETL Using Core Python
- HTML5 and CSS3: Craft your own websites (with 4 projects)
- The Complete Adalo App Beginners Course ( Apps With Nocode)
- Python and DB2 App Development: Build a CRUD Application
- Supervised Machine Learning in Python
- MERN Stack Doctor Appointment Booking App
- Build Role-based Authentication using Node.js, JWT, MongoDB
- Python Complete Course: with 30+ Hands-on Tasks and Solution
- SQL Bootcamp – Hands-On Exercises – SQLite – Part II
- Build a Data Science web app using Streamlit
- Product Management 101: Essential Skills for Success
- C++ Practice Tests: Complete Coverage of C++ Topics