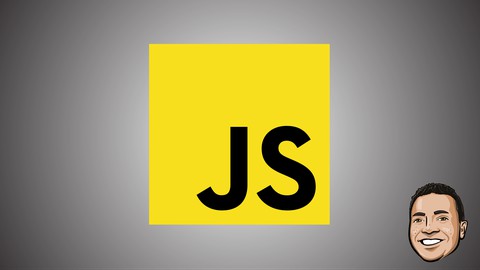
JavaScript: Understanding the Weird Parts (2024 Edition)
JavaScript: Understanding the Weird Parts (2024 Edition), available at $129.99, has an average rating of 4.67, with 107 lectures, based on 48289 reviews, and has 192773 subscribers.
You will learn about Grasp how Javascript works and it's fundamental concepts Write solid, good Javascript code Understand advanced concepts such as closures, prototypal inheritance, IIFEs, and much more. Drastically improve your ability to debug problems in Javascript. Avoid common pitfalls and mistakes other Javascript coders make Understand the source code of popular Javascript frameworks Build your own Javascript framework or library This course is ideal for individuals who are Those with basic Javascript skills who wish to improve or Experienced coders coming from other programming languages or New and experienced Javascript coders who want to deepen their understanding of the language or Anyone who has found concepts just as object prototypes, closures, and other advanced concepts difficult to learn or Those who have suffered surprising errors while writing Javascript, and want to learn why and how to avoid them or Those interested in building their own frameworks, or being better able to learn from the source code of other well-known frameworks and libraries It is particularly useful for Those with basic Javascript skills who wish to improve or Experienced coders coming from other programming languages or New and experienced Javascript coders who want to deepen their understanding of the language or Anyone who has found concepts just as object prototypes, closures, and other advanced concepts difficult to learn or Those who have suffered surprising errors while writing Javascript, and want to learn why and how to avoid them or Those interested in building their own frameworks, or being better able to learn from the source code of other well-known frameworks and libraries.
Enroll now: JavaScript: Understanding the Weird Parts (2024 Edition)
Summary
Title: JavaScript: Understanding the Weird Parts (2024 Edition)
Price: $129.99
Average Rating: 4.67
Number of Lectures: 107
Number of Published Lectures: 92
Number of Curriculum Items: 107
Number of Published Curriculum Objects: 92
Original Price: $159.99
Quality Status: approved
Status: Live
What You Will Learn
- Grasp how Javascript works and it's fundamental concepts
- Write solid, good Javascript code
- Understand advanced concepts such as closures, prototypal inheritance, IIFEs, and much more.
- Drastically improve your ability to debug problems in Javascript.
- Avoid common pitfalls and mistakes other Javascript coders make
- Understand the source code of popular Javascript frameworks
- Build your own Javascript framework or library
Who Should Attend
- Those with basic Javascript skills who wish to improve
- Experienced coders coming from other programming languages
- New and experienced Javascript coders who want to deepen their understanding of the language
- Anyone who has found concepts just as object prototypes, closures, and other advanced concepts difficult to learn
- Those who have suffered surprising errors while writing Javascript, and want to learn why and how to avoid them
- Those interested in building their own frameworks, or being better able to learn from the source code of other well-known frameworks and libraries
Target Audiences
- Those with basic Javascript skills who wish to improve
- Experienced coders coming from other programming languages
- New and experienced Javascript coders who want to deepen their understanding of the language
- Anyone who has found concepts just as object prototypes, closures, and other advanced concepts difficult to learn
- Those who have suffered surprising errors while writing Javascript, and want to learn why and how to avoid them
- Those interested in building their own frameworks, or being better able to learn from the source code of other well-known frameworks and libraries
Javascript is the language that modern developers need to know, and know well. Truly knowing Javascript will get you a job, and enable you to build quality web and server applications.
NOTE: This course includes information on ECMAScript 6 (ES6) the next version of Javascript!
In this course you will gain a deep understanding of Javascript, learn how Javascript works under the hood, and how that knowledge helps you avoid common pitfalls and drastically improve your ability to debug problems. You will find clarity in the parts that others, even experienced coders, may find weird, odd, and at times incomprehensible. You'll learn the beauty and deceptive power of this language that is at the forefront of modern software development today.
This course will cover such advanced concepts as objects and object literals, function expressions, prototypical inheritance, functional programming, scope chains, function constructors (plus new ES6 features), immediately invoked function expressions (IIFEs), call, apply, bind, and more.
We'll take a deep dive into the source code of popular frameworks such as jQuery and Underscore to see how you can use your understanding of Javascript to learn (and borrow) from other's good code.
Finally, you'll learn the foundations of how to build your own Javascript framework or library.
What you'll learn in this course will make you a better Javascript developer, and improve your abilities in AngularJS, NodeJS, jQuery, React, Ember, MongoDB, and all other Javascript-based technologies!
Learn to love Javascript, and code in it well.
Note: In this course you'll also get downloadable source code. You will often be provided with 'starter' code, giving you the base for you to start writing your code, and 'finished' code to compare your code to.
Course Curriculum
Chapter 1: Getting Started
Lecture 1: Introduction and The Goal of This Course
Lecture 2: Setup
Lecture 3: Setup (Visual Studio Code)
Lecture 4: Big Words and Javascript
Lecture 5: Watching this Course in High Definition
Lecture 6: Understanding, Frameworks, and The Weird Parts
Chapter 2: Execution Contexts and Lexical Environments
Lecture 1: Conceptual Aside: Syntax Parsers, Execution Contexts, and Lexical Environments
Lecture 2: Conceptual Aside: Name/Value Pairs and Objects
Lecture 3: Downloading Source Code for This Course
Lecture 4: The Global Environment and The Global Object
Lecture 5: The Execution Context – Creation and Hoisting
Lecture 6: Conceptual Aside: Javascript and 'undefined'
Lecture 7: The Execution Context – Code Execution
Lecture 8: Conceptual Aside: Single Threaded, Synchronous Execution
Lecture 9: Function Invocation and the Execution Stack
Lecture 10: Functions, Context, and Variable Environments
Lecture 11: The Scope Chain
Lecture 12: Scope, ES6, and let
Lecture 13: What About Asynchronous Callbacks?
Chapter 3: Types and Operators
Lecture 1: Conceptual Aside: Types and Javascript
Lecture 2: Primitive Types
Lecture 3: Conceptual Aside: Operators
Lecture 4: Operator Precedence and Associativity
Lecture 5: Operator Precedence and Associativity Table
Lecture 6: Conceptual Aside: Coercion
Lecture 7: Comparison Operators
Lecture 8: Equality Comparisons Table
Lecture 9: Existence and Booleans
Lecture 10: Default Values
Lecture 11: Framework Aside: Default Values
Chapter 4: Objects and Functions
Lecture 1: Objects and the Dot
Lecture 2: Objects and Object Literals
Lecture 3: Framework Aside: Faking Namespaces
Lecture 4: JSON and Object Literals
Lecture 5: Functions are Objects
Lecture 6: Function Statements and Function Expressions
Lecture 7: Conceptual Aside: By Value vs By Reference
Lecture 8: Objects, Functions, and 'this'
Lecture 9: Conceptual Aside: Arrays – Collections of Anything
Lecture 10: 'arguments' and spread
Lecture 11: Framework Aside: Function Overloading
Lecture 12: Conceptual Aside: Syntax Parsers
Lecture 13: Dangerous Aside: Automatic Semicolon Insertion
Lecture 14: Framework Aside: Whitespace
Lecture 15: Immediately Invoked Functions Expressions (IIFEs)
Lecture 16: Framework Aside: IIFEs and Safe Code
Lecture 17: Understanding Closures
Lecture 18: Understanding Closures – Part 2
Lecture 19: Framework Aside: Function Factories
Lecture 20: Closures and Callbacks
Lecture 21: call(), apply(), and bind()
Lecture 22: Functional Programming
Lecture 23: Functional Programming – Part 2
Chapter 5: Object-Oriented Javascript and Prototypal Inheritance
Lecture 1: Conceptual Aside: Classical vs Prototypal Inheritance
Lecture 2: Understanding the Prototype
Lecture 3: Everything is an Object (or a primitive)
Lecture 4: Reflection and Extend
Chapter 6: Building Objects
Lecture 1: Function Constructors, 'new', and the History of Javascript
Lecture 2: Function Constructors and '.prototype'
Lecture 3: Dangerous Aside: 'new' and functions
Lecture 4: Conceptual Aside: Built-In Function Constructors
Lecture 5: Dangerous Aside: Built-In Function Constructors
Lecture 6: Dangerous Aside: Arrays and for..in
Lecture 7: Object.create and Pure Prototypal Inheritance
Lecture 8: ES6 and Classes
Chapter 7: Odds and Ends
Lecture 1: Initialization
Lecture 2: 'typeof' , 'instanceof', and Figuring Out What Something Is
Lecture 3: Strict Mode
Lecture 4: Strict Mode Reference
Chapter 8: Examining Famous Frameworks and Libraries
Lecture 1: Learning From Other's Good Code
Lecture 2: Deep Dive into Source Code: jQuery – Part 1
Lecture 3: Deep Dive into Source Code: jQuery – Part 2
Lecture 4: Deep Dive into Source Code: jQuery – Part 3
Chapter 9: Let's Build a Framework / Library!
Lecture 1: Requirements
Lecture 2: Structuring Safe Code
Lecture 3: Our Object and Its Prototype
Lecture 4: Properties and Chainable Methods
Lecture 5: Adding jQuery Support
Lecture 6: Good Commenting
Lecture 7: Let's Use Our Framework
Lecture 8: A Side Note
Chapter 10: EXTRA: TypeScript, ES6, and Transpiled Languages
Lecture 1: TypeScript, ES6, and Transpiled Languages
Lecture 2: Transpiled Languages References
Chapter 11: Promises, Async, and Await
Lecture 1: About This Section
Lecture 2: Promises, Async, and Await
Chapter 12: ES6: The Most Used Parts
Lecture 1: ES6: The Most Used Parts
Lecture 2: let
Lecture 3: const
Instructors
-
Anthony Alicea
Software Developer, Architect, and UX Designer
Rating Distribution
- 1 stars: 195 votes
- 2 stars: 340 votes
- 3 stars: 1849 votes
- 4 stars: 10553 votes
- 5 stars: 35346 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Best Yoga Instruction Courses to Learn in March 2025
- Best Stress Management Courses to Learn in March 2025
- Best Mindfulness Meditation Courses to Learn in March 2025
- Best Life Coaching Courses to Learn in March 2025
- Best Career Development Courses to Learn in March 2025
- Best Relationship Building Courses to Learn in March 2025
- Best Parenting Skills Courses to Learn in March 2025
- Best Home Improvement Courses to Learn in March 2025
- Best Gardening Courses to Learn in March 2025
- Best Sewing And Knitting Courses to Learn in March 2025
- Best Interior Design Courses to Learn in March 2025
- Best Writing Courses Courses to Learn in March 2025
- Best Storytelling Courses to Learn in March 2025
- Best Creativity Workshops Courses to Learn in March 2025
- Best Resilience Training Courses to Learn in March 2025
- Best Emotional Intelligence Courses to Learn in March 2025
- Best Time Management Courses to Learn in March 2025
- Best Remote Work Strategies Courses to Learn in March 2025
- Best Freelancing Courses to Learn in March 2025
- Best E-commerce Strategies Courses to Learn in March 2025