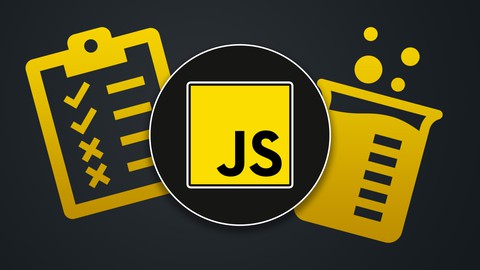
JavaScript Unit Testing – The Practical Guide
JavaScript Unit Testing – The Practical Guide, available at $99.99, has an average rating of 4.56, with 94 lectures, based on 4257 reviews, and has 26525 subscribers.
You will learn about Write and structure unit & integration tests Remove side effects from tests via mocking and spies Work with popular JavaScript test runners and libraries Write good tests and focus on testing core business logic This course is ideal for individuals who are JavaScript developers who want to add tests to their projects or Developers who always considered automated testing to be intimidating It is particularly useful for JavaScript developers who want to add tests to their projects or Developers who always considered automated testing to be intimidating.
Enroll now: JavaScript Unit Testing – The Practical Guide
Summary
Title: JavaScript Unit Testing – The Practical Guide
Price: $99.99
Average Rating: 4.56
Number of Lectures: 94
Number of Published Lectures: 93
Number of Curriculum Items: 94
Number of Published Curriculum Objects: 93
Original Price: $124.99
Quality Status: approved
Status: Live
What You Will Learn
- Write and structure unit & integration tests
- Remove side effects from tests via mocking and spies
- Work with popular JavaScript test runners and libraries
- Write good tests and focus on testing core business logic
Who Should Attend
- JavaScript developers who want to add tests to their projects
- Developers who always considered automated testing to be intimidating
Target Audiences
- JavaScript developers who want to add tests to their projects
- Developers who always considered automated testing to be intimidating
Automated testing is a key conceptin modern (web) development.
It is a concept that can be intimidating at first, hence many developers shy away from diving into testing and adding tests to their projects.
This course teaches you automated unit & integration testing with JavaScript from the ground up. You will learn how tests are written and added to your projects, what should (and should not) be tested and how you can test both simple as well as more complex code.
You will learn about the software and setup required to write automated tests and example projects will be provided as part of the course. It’s a hands-on, practical course, hence you won’t get stuck in theory – instead you’ll be able to learn all key concepts at real examples.
In the course, Vitest will be used as the main testing library & tool. It’s a modern JavaScript test runner and assertion library that provides Jest compatibility. Hence what you’ll learn in this course will help you no matter if you’re working with Vitest or Jest. And the core concepts will apply, no matter which testing setup you’re using at all!
As part of this course, typical testing problems will be defined and solved and common strategies like mocking or working with spies are taught in great detail. This course also does not focus on specific types of JavaScript projects – neither does it focus on any specific library or framework.
Instead, you’ll learn how to automatically test your (vanilla) JavaScript code, no matter if it’s a NodeJS or frontend project. The fundamentals you’ll gain in this course will help you in all your future projects – backend (NodeJS) and frontend (vanilla JS, React, Vue, Angular) alike.
This course will provide you with an extremely solid foundation to build up on, such that you can start adding tests to all your JavaScript projects.
In detail, this course will teach you:
-
What exactly “testing” or “automated testing” is (and why you need it)
-
What “unit testing” is specifically
-
Which tools you need to enable automated unit tests in your projects
-
How to write unit tests
-
How to get started with integration tests
-
How to formulate different expectations (assertions)
-
Which patterns to follow when writing tests
-
How to test asynchronous and synchronous code
-
How to deal with side effects with help of spies & mocks
-
How to apply all these concepts in real projects & examples
Course Curriculum
Chapter 1: Getting Started
Lecture 1: Welcome To This Course!
Lecture 2: What Is Testing?
Lecture 3: Unit Testing: What & Why?
Lecture 4: Unit vs Integration vs E2E Tests
Lecture 5: A Quick Note About Test-Driven Development (TDD)
Lecture 6: About This Course
Lecture 7: Join Our Learning Community
Lecture 8: Course Resources & Project Snapshots
Lecture 9: Course Setup
Chapter 2: Setup & Testing Software
Lecture 1: Module Introduction
Lecture 2: Which Tools Are Needed For Testing?
Lecture 3: Jest & Vitest
Lecture 4: Installing Vitest
Lecture 5: Course Project Setup
Chapter 3: Testing Basics
Lecture 1: Module Introduction
Lecture 2: Basic Test File & Project Setup
Lecture 3: Writing a First Test
Lecture 4: Running Tests
Lecture 5: Why Are We Testing?
Lecture 6: The AAA Pattern – Arrange, Act, Assert
Lecture 7: Keep Your Tests Simple!
Lecture 8: Defining Behaviors & Fixing Errors In Your Code
Lecture 9: Demo: Writing More Tests
Lecture 10: Testing For Errors
Lecture 11: Demo: Adding More Tests
Lecture 12: Testing For Thrown Errors & Error Messages
Lecture 13: Exercise: Problem
Lecture 14: Exercise: Solution
Lecture 15: Tests With Multiple Assertions (Multiple Expectations)
Lecture 16: More Practice!
Lecture 17: Introducing Test Suites
Lecture 18: Adding Tests To Frontend & Backend Projects
Lecture 19: Module Summary
Chapter 4: Writing Good Tests
Lecture 1: Module Introduction
Lecture 2: What To Test & Not To Test
Lecture 3: Writing Good Tests – An Overview & Summary
Lecture 4: Only Test "One Thing"
Lecture 5: Splitting Functions For Easier Testing & Better Code
Lecture 6: Refactoring Code
Lecture 7: Formulating Different Expectations
Lecture 8: A Word About Code Coverage
Lecture 9: Module Summary
Chapter 5: Integration Tests
Lecture 1: Module Introduction
Lecture 2: Introducing Integration Tests
Lecture 3: Writing an Integration Test & Reasoning
Lecture 4: Testing For Errors
Lecture 5: Integration vs Unit Tests: Finding the Right Balance
Chapter 6: Advanced Testing Concepts
Lecture 1: Module Introduction
Lecture 2: toBe() vs toEqual()
Lecture 3: The Problem With Asynchronous Code
Lecture 4: Testing Asynchronous Code With Callbacks
Lecture 5: Testing Asynchronous Code With Promises & async / await
Lecture 6: Returning Promises In Tests
Lecture 7: Getting Started with "Testing Hooks"
Lecture 8: Why Hooks?
Lecture 9: Using Testing Hooks (beforeEach, beforeAll, afterEach, afterAll)
Lecture 10: Concurrent Tests
Lecture 11: Concurrency & Default Behavior
Lecture 12: Module Summary
Chapter 7: Mocking & Spies: Dealing with Side Effects
Lecture 1: Module Introduction
Lecture 2: The Starting Project
Lecture 3: The Problem With Side Effects & Tests
Lecture 4: Introducing Spies & Mocks
Lecture 5: Working with Spies
Lecture 6: Getting Started with Mocks & Automocking
Lecture 7: Note on Mocking
Lecture 8: Notes on Spies
Lecture 9: Custom Mocking Logic
Lecture 10: Managing Custom Mock Implementations Globally (__mocks__ Folder)
Lecture 11: More Mocking Functionalities
Lecture 12: Module Summary
Chapter 8: More on Mocking & Diving Deeper
Lecture 1: Module Introduction
Lecture 2: The Starting Project
Lecture 3: Refresher: Practicing Basic Tests (1)
Lecture 4: Refresher: Practicing Basic Tests (2)
Lecture 5: Reasons to Mock
Lecture 6: Mocking Global Values & Functions
Lecture 7: Mocking Frontend Libraries
Lecture 8: Test-specific Mocking Logic
Lecture 9: Mocking In Selected Places
Lecture 10: Using Local Mock Values
Lecture 11: Module Summary
Chapter 9: Testing & The DOM (Frontend JavaScript Testing)
Lecture 1: Module Introduction
Lecture 2: Understanding the Problem
Lecture 3: Working with Different Testing Environments
Lecture 4: Setting up a Virtual HTML Page
Lecture 5: Testing DOM Functionalities
Lecture 6: DOM Tests & Cleanup Work
Lecture 7: Finishing Example
Lecture 8: A Brief Look at the "Testing Library" Package
Lecture 9: Module Summary
Instructors
-
Academind by Maximilian Schwarzmüller
Online Education -
Maximilian Schwarzmüller
AWS certified, Professional Web Developer and Instructor
Rating Distribution
- 1 stars: 17 votes
- 2 stars: 25 votes
- 3 stars: 223 votes
- 4 stars: 1314 votes
- 5 stars: 2678 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple