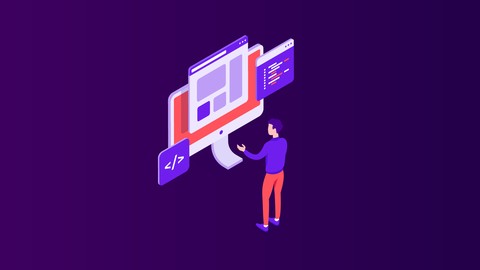
Learning Path: Modern Web Development with JavaScript
Learning Path: Modern Web Development with JavaScript, available at $19.99, has an average rating of 4.11, with 148 lectures, 18 quizzes, based on 9 reviews, and has 109 subscribers.
You will learn about Understand key principles such as object inheritance and the correct usage of JavaScript mixins Master dynamic typing and polymorphism with examples that reflect key implementation challenges Master dynamic typing and polymorphism with examples that reflect key implementation challenges Use WebRTC APIs and the WebSocket protocol for browser-based video communication Utilize the Bacon. js library for both server-side and frontend development Build an example application UI with React and Flux Understand asynchronous programming with Node .js Develop scalable and high-performing APIs using hapi .js and Knex .js This course is ideal for individuals who are This is an ideal Learning Path for you, if you are a programmer who is new to JavaScript, or have entry-level JavaScript experience, or are already well-versed in JavaScript. You'll find plenty of demonstrations and guided demos that are designed to build upon your existing skills. or Though prior experience with other server-side technologies such as Python, PHP, ASP .NET, Ruby, and NoSQL databases such as MongoDB will help, it’s not essential to have a background in backend development before getting started. It is particularly useful for This is an ideal Learning Path for you, if you are a programmer who is new to JavaScript, or have entry-level JavaScript experience, or are already well-versed in JavaScript. You'll find plenty of demonstrations and guided demos that are designed to build upon your existing skills. or Though prior experience with other server-side technologies such as Python, PHP, ASP .NET, Ruby, and NoSQL databases such as MongoDB will help, it’s not essential to have a background in backend development before getting started.
Enroll now: Learning Path: Modern Web Development with JavaScript
Summary
Title: Learning Path: Modern Web Development with JavaScript
Price: $19.99
Average Rating: 4.11
Number of Lectures: 148
Number of Quizzes: 18
Number of Published Lectures: 148
Number of Published Quizzes: 18
Number of Curriculum Items: 166
Number of Published Curriculum Objects: 166
Original Price: $199.99
Quality Status: approved
Status: Live
What You Will Learn
- Understand key principles such as object inheritance and the correct usage of JavaScript mixins
- Master dynamic typing and polymorphism with examples that reflect key implementation challenges
- Master dynamic typing and polymorphism with examples that reflect key implementation challenges
- Use WebRTC APIs and the WebSocket protocol for browser-based video communication
- Utilize the Bacon. js library for both server-side and frontend development
- Build an example application UI with React and Flux
- Understand asynchronous programming with Node .js
- Develop scalable and high-performing APIs using hapi .js and Knex .js
Who Should Attend
- This is an ideal Learning Path for you, if you are a programmer who is new to JavaScript, or have entry-level JavaScript experience, or are already well-versed in JavaScript. You'll find plenty of demonstrations and guided demos that are designed to build upon your existing skills.
- Though prior experience with other server-side technologies such as Python, PHP, ASP .NET, Ruby, and NoSQL databases such as MongoDB will help, it’s not essential to have a background in backend development before getting started.
Target Audiences
- This is an ideal Learning Path for you, if you are a programmer who is new to JavaScript, or have entry-level JavaScript experience, or are already well-versed in JavaScript. You'll find plenty of demonstrations and guided demos that are designed to build upon your existing skills.
- Though prior experience with other server-side technologies such as Python, PHP, ASP .NET, Ruby, and NoSQL databases such as MongoDB will help, it’s not essential to have a background in backend development before getting started.
We ease you into the world of JavaScript and Node.js with an introduction to their fundamental concepts. We’ll show you everything you need to know about object-oriented patterns so that you can confidently tackle your own real-world development projects. You’ll learn everything from new syntax to working with classes, complex inheritance, dynamic typing, and data binding. Then, we will take a look at the libraries in JavaScript that aid in building applications with a microservices-based architecture. We will look at building these applications and explore a number of industry-standard best practices. With coverage of both server-side and front-end development, this Learning Path provides you the skills required to develop cutting-edge web applications that stand the test of time. We’ll demonstrate the creation of an example client that pairs up with a fully authenticated API implementation. By the end of this Learning Path, you’ll have the skills and exposure for building interactive web applications that use object-oriented patterns with JavaScript and APIs with Node .js.
Course Curriculum
Chapter 1: Beginning Object-Oriented Programming with JavaScript
Lecture 1: Course Overview
Lecture 2: Lesson Overview
Lecture 3: Creating and Managing Object Literals
Lecture 4: Properties
Lecture 5: Methods
Lecture 6: Defining Object Constructors
Lecture 7: Using Object Prototypes
Lecture 8: Using Classes
Lecture 9: Beginning with Object-Oriented JavaScript
Lecture 10: Checking Abstraction and Modeling Support
Lecture 11: Association
Lecture 12: Aggregation
Lecture 13: Composition
Lecture 14: Analyzing OOP Principle Support in JavaScript
Lecture 15: Polymorphism
Lecture 16: JavaScript OOP versus Classical OOP
Lecture 17: Summary
Lecture 18: Lesson Overview
Lecture 19: Encapsulation and Information Hiding
Lecture 20: Privacy Levels
Lecture 21: Using the Meta-Closure Approach
Lecture 22: Managing Isolated Private Members
Lecture 23: A Definitive Solution with WeakMap
Lecture 24: Using Property Descriptors Part-1
Lecture 25: Using Property Descriptors Part-2
Lecture 26: Implementing Information Hiding in ES6 Classes
Lecture 27: Lesson Summary
Lecture 28: Lesson Overview
Lecture 29: Implementing Inheritance
Lecture 30: Objects and Prototypes
Lecture 31: Prototype Chaining
Lecture 32: Inheritance and Constructors
Lecture 33: Using Class Inheritance
Lecture 34: Overriding Methods
Lecture 35: Overriding Properties
Lecture 36: Protected Members
Lecture 37: Implementing Multiple Inheritance
Lecture 38: Creating and Using Mixins
Lecture 39: Lesson Summary
Lecture 40: Lesson Overview
Lecture 41: Managing Dynamic Typing
Lecture 42: Dynamic Data Types
Lecture 43: Beyond the Instance Type
Lecture 44: Contracts and Interfaces
Lecture 45: Implementing Duck Typing
Lecture 46: Defining a Private Function
Lecture 47: A General Solution
Lecture 48: Emulating Interfaces with Duck Typing
Lecture 49: Demonstrating the Equivalent Version without ECMAScript 2015 Syntax
Lecture 50: Multiple Interface Implementation
Lecture 51: Comparing Duck Typing and Polymorphism
Lecture 52: Lesson Summary
Lecture 53: Lesson Overview
Lecture 54: Creating Objects
Lecture 55: Creating a Singleton
Lecture 56: Mysterious Behavior of Constructors
Lecture 57: Singletons
Lecture 58: Implementing an Object Factory
Lecture 59: The Abstract Factory
Lecture 60: The Builder Pattern
Lecture 61: Lesson Summary
Lecture 62: Lesson Overview
Lecture 63: Managing User Interfaces
Lecture 64: Implementing Presentation Patterns
Lecture 65: The Model-View-Controller Pattern
Lecture 66: The Model-View-Presenter Pattern
Lecture 67: The Model-View-ViewModel Pattern
Lecture 68: Data Binding
Lecture 69: Implementing Data Binding
Lecture 70: Monitoring Changes
Lecture 71: Hacking Properties
Lecture 72: Setting up a Data Binding Relationship
Lecture 73: Applying the Publish/Subscribe Pattern
Lecture 74: Lesson Summary
Lecture 75: Lesson Overview
Lecture 76: Event Loop and Asynchronous Code
Lecture 77: Events, Ajax, and Other Asynchronous Stuff
Lecture 78: Writing Asynchronous Code
Lecture 79: Issues of Asynchronous Code
Lecture 80: Promises
Lecture 81: Lesson Summary
Lecture 82: Lesson Overview
Lecture 83: Taking Control of the Global Scope
Lecture 84: Creating Namespaces
Lecture 85: Organizing Code with the Module Pattern
Lecture 86: Augmentation
Lecture 87: Composing Modules
Lecture 88: Loading the Module
Lecture 89: Module Loader Issues
Lecture 90: Asynchronous Module Definition
Lecture 91: Using the ECMAScript 2015 Modules
Lecture 92: Lesson Summary
Instructors
-
Packt Publishing
Tech Knowledge in Motion
Rating Distribution
- 1 stars: 0 votes
- 2 stars: 1 votes
- 3 stars: 1 votes
- 4 stars: 5 votes
- 5 stars: 2 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Top 10 Video Editing Courses to Learn in November 2024
- Top 10 Music Production Courses to Learn in November 2024
- Top 10 Animation Courses to Learn in November 2024
- Top 10 Digital Illustration Courses to Learn in November 2024
- Top 10 Renewable Energy Courses to Learn in November 2024
- Top 10 Sustainable Living Courses to Learn in November 2024
- Top 10 Ethical AI Courses to Learn in November 2024
- Top 10 Cybersecurity Fundamentals Courses to Learn in November 2024
- Top 10 Smart Home Technology Courses to Learn in November 2024
- Top 10 Holistic Health Courses to Learn in November 2024
- Top 10 Nutrition And Diet Planning Courses to Learn in November 2024
- Top 10 Yoga Instruction Courses to Learn in November 2024
- Top 10 Stress Management Courses to Learn in November 2024
- Top 10 Mindfulness Meditation Courses to Learn in November 2024
- Top 10 Life Coaching Courses to Learn in November 2024
- Top 10 Career Development Courses to Learn in November 2024
- Top 10 Relationship Building Courses to Learn in November 2024
- Top 10 Parenting Skills Courses to Learn in November 2024
- Top 10 Home Improvement Courses to Learn in November 2024
- Top 10 Gardening Courses to Learn in November 2024