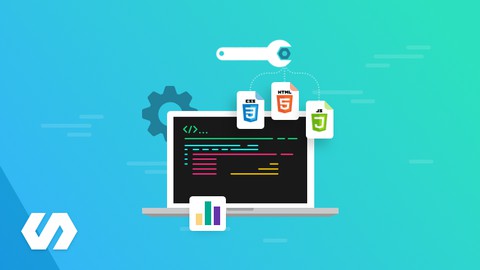
NestJS: The Complete Developer's Guide
NestJS: The Complete Developer's Guide, available at $94.99, has an average rating of 4.66, with 249 lectures, based on 7175 reviews, and has 41884 subscribers.
You will learn about Deploy a feature-complete app to production Build authentication and permissions systems from scratch Write integration and unit tests to ensure your code is working Automatically validate data included with incoming requests Use an API client to manually test your app Apply structure to your code with Typescript Make your code more reusable and testable with dependency injection Tie different types of data together with TypeORM relationships Get a behind-the-scenes understanding of NestJS Use Guards to prevent unauthorized users from gaining access to sensitive data Understand Nest's special request-response cycle Model your app's data using TypeORM entities Use decorators to dramatically simplify your code This course is ideal for individuals who are Any engineer looking to build an API It is particularly useful for Any engineer looking to build an API.
Enroll now: NestJS: The Complete Developer's Guide
Summary
Title: NestJS: The Complete Developer's Guide
Price: $94.99
Average Rating: 4.66
Number of Lectures: 249
Number of Published Lectures: 244
Number of Curriculum Items: 249
Number of Published Curriculum Objects: 244
Original Price: $199.99
Quality Status: approved
Status: Live
What You Will Learn
- Deploy a feature-complete app to production
- Build authentication and permissions systems from scratch
- Write integration and unit tests to ensure your code is working
- Automatically validate data included with incoming requests
- Use an API client to manually test your app
- Apply structure to your code with Typescript
- Make your code more reusable and testable with dependency injection
- Tie different types of data together with TypeORM relationships
- Get a behind-the-scenes understanding of NestJS
- Use Guards to prevent unauthorized users from gaining access to sensitive data
- Understand Nest's special request-response cycle
- Model your app's data using TypeORM entities
- Use decorators to dramatically simplify your code
Who Should Attend
- Any engineer looking to build an API
Target Audiences
- Any engineer looking to build an API
Authentication/Authorization? Covered. Automated Testing?Yep, it’s here! Production Deployment?Of course!
Congratulations! You’ve found the complete guide on how to build enterprise-ready apps with NestJS.
NestJS is a backend framework used to create scalable and reliable APIs. It is a “battery-included” framework; it includes tools to handle just about every possible use case, from data persistence, to validation, to config management, to testing, and much, much more. This course will help you master Nest. By the time you complete this course, you will have the confidence to build any app you can imagine.
Throughout this course you will build a series of apps with growing complexity. We use as few libraries and tools as possible. Instead, you will write many custom systems to better understand how every piece of Nest works together. Each application you build includes discussion on data modeling and persistence. We will first save records in a simple file-based data store (built from scratch) and eventually work our way up to saving data in a production-grade Postgres instance.
Testing is a fundamental topic in Nest. A tremendous amount of functionality in Nest is dedicated to making sure your project is easy to test. This course follows Nest’s testing recommendations, and you will write both integration and unit tests to ensure your project is working as expected. Although testing can sometimes be confusing and boring, I have put special care into making sure the tests we write are expressive, fast, and effective. You will be able to use this knowledge on your own projects, even those that don’t use Nest!
Typescript is used throughout this course to make sure we are writing clean and correct code.Don’t know Typescript? Not a problem! A free appendix is included at the end of the course to get you up to speed on Typescript. Once you’re familiar with it, Typescript will help you catch errors and bugs in your code before you even run it. If you’ve never used Typescript before you are in for a treat 🙂
————————————–
Everything in this course is designed to make your learning process as easy as possible.
-
At every step, I will teach you what Nest is doing internally, and help you understand how to twist and bend Nest to better suit your application’s needs.
-
Every single video in the course has an attached ZIP file containing up-to-date code, just in case you ever get stuck.
-
Full-time teaching assistants are standing by to help answer your questions
-
Access private live chat server is included. Live help whenever you need it!
————————————–
Here’s a partial list of the topics included in this course:
-
Securely deploy your app to production
-
Write automated integration and unit tests to make sure your code is working
-
Build an authentication system from scratch to log users in
-
Allow users to perform certain actions with a permissions system
-
Store and retrieve data with complex queries using TypeORM
-
Understand how TypeORM handles data relationships
-
Write declarative code using property, method, and parameter decorators
-
Master the concept of dependency injectionto write reusable code
-
Implement automatic validation of incoming requests
-
Format outgoing response data with a custom DTO system
-
Handle incoming requests and outgoing responses using Guards and Interceptors
-
Segment your code into reusable Nest Modules
-
Add structure to your database using migrations
I had a tough time learning NestJS. There are a tremendous number of outdated tutorials around it, the documentation is sometimes unclear, and Nest itself is just plain hard to understand. I made this course to save you time and money – a course to show you exactly what you need to know about every topic in Nest. You will find learning Nest to be a delightful experience and pick up a tremendous amount of knowledge along the way.
Sign up today and join me in mastering NestJS!
Course Curriculum
Chapter 1: Get Started Here!
Lecture 1: How to Get Help
Lecture 2: Join Our Community!
Lecture 3: Course Resources
Chapter 2: The Basics of Nest
Lecture 1: Project Setup
Lecture 2: TypeScript Configuration
Lecture 3: Creating a Controller
Lecture 4: Starting Up a Nest App
Lecture 5: File Naming Conventions
Lecture 6: Routing Decorators
Chapter 3: Generating Projects with the Nest CLI
Lecture 1: App Setup
Lecture 2: Using the Nest CLI to Generate Files
Lecture 3: More on Generating Files
Lecture 4: Adding Routing Logic
Lecture 5: [Optional] Postman Setup
Lecture 6: [Optional] VSCode REST Client Extension
Chapter 4: Validating Request Data with Pipes
Lecture 1: Accessing Request Data with Decorators
Lecture 2: Installing Extra Required Libraries
Lecture 3: Using Pipes for Validation
Lecture 4: Adding Validation Rules
Lecture 5: Behind the Scenes of Validation
Lecture 6: How Type Info is Preserved
Chapter 5: Nest Architecture: Services and Repositories
Lecture 1: Services and Repositories
Lecture 2: Implementing a Repository
Lecture 3: Reading and Writing to a Storage File
Lecture 4: Implementing a Service
Lecture 5: Manual Testing of the Controller
Lecture 6: Reporting Errors with Exceptions
Lecture 7: Understanding Inversion of Control
Lecture 8: Introduction to Dependency Injection
Lecture 9: Refactoring to Use Dependency Injection
Lecture 10: Few More Notes on DI
Chapter 6: Nest Architecture: Organizing Code with Modules
Lecture 1: Project Overview
Lecture 2: Generating a Few Files
Lecture 3: Setting Up DI Between Modules
Lecture 4: More on DI Between Modules
Lecture 5: Consuming Multiple Modules
Lecture 6: Modules Wrapup
Chapter 7: Big Project Time!
Lecture 1: App Overview
Lecture 2: API Design
Lecture 3: Module Design!
Lecture 4: Generating Modules, Controllers, and Services
Chapter 8: Persisting Data with TypeORM
Lecture 1: Persistent Data with Nest
Lecture 2: Setting Up a Database Connection
Lecture 3: Creating an Entity and Repository
Lecture 4: Viewing a DB's Contents
Lecture 5: Understanding TypeORM Decorators
Lecture 6: One Quick Note on Repositories
Lecture 7: A Few Extra Routes
Lecture 8: Setting Up Body Validation
Lecture 9: Manual Route Testing
Chapter 9: Creating and Saving User Data
Lecture 1: Creating and Saving a User
Lecture 2: Quick Breather and Review
Lecture 3: More on Create vs Save
Lecture 4: Required Update for find and findOne Methods
Lecture 5: Querying for Data
Lecture 6: Updating Data
Lecture 7: Removing Users
Lecture 8: Finding and Filtering Records
Lecture 9: Removing Records
Lecture 10: Updating Records
Lecture 11: A Few Notes on Exceptions
Chapter 10: Custom Data Serialization
Lecture 1: Excluding Response Properties
Lecture 2: Solution to Serialization
Lecture 3: How to Build Interceptors
Lecture 4: Serialization in the Interceptor
Lecture 5: Customizing the Interceptor's DTO
Lecture 6: Wrapping the Interceptor in a Decorator
Lecture 7: Controller-Wide Serialization
Lecture 8: A Bit of Type Safety Around Serialize
Chapter 11: Authentication From Scratch
Lecture 1: Authentication Overview
Lecture 2: Reminder on Service Setup
Lecture 3: Implementing Signup Functionality
Lecture 4: [Optional] Understanding Password Hashing
Lecture 5: Salting and Hashing the Password
Lecture 6: Creating a User
Lecture 7: Handling User Sign In
Lecture 8: Setting up Sessions
Lecture 9: Changing and Fetching Session Data
Lecture 10: Signing in a User
Lecture 11: Getting the Current User
Lecture 12: Signing Out a User
Lecture 13: Two Automation Tools
Lecture 14: Custom Param Decorators
Lecture 15: Why a Decorator and Interceptor
Lecture 16: Communicating from Interceptor to Decorator
Lecture 17: Small Fix for CurrentUserInterceptor
Lecture 18: Connecting an Interceptor to Dependency Injection
Lecture 19: Globally Scoped Interceptors
Lecture 20: Preventing Access with Authentication Guards
Instructors
-
Stephen Grider
Engineering Architect
Rating Distribution
- 1 stars: 32 votes
- 2 stars: 59 votes
- 3 stars: 286 votes
- 4 stars: 1738 votes
- 5 stars: 5067 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Complete Python Course: Go from zero to hero!
- Introduction to YAML – A hands-on course
- NestJS: The Complete Developer's Guide
- Build A TodoList with Kotlin, Spring Boot and React
- Object Oriented Programming with Java
- Game Development: Basic Games with C# and Unity 3D
- Flutter Artificial Intelligence Course – Build 15+ AI Apps
- Complete Unity C# & Android game development Bootcamp 2020
- Building Applications Using Java and NetBeans
- Build Real World App In Xamarin Forms
- 3D Game Development with GameGuru
- PHP for Beginners: PHP Crash Course
- Blazor Crash Course for Absolute Beginners
- Control a LED Matrix via web interface with Arduino ESP32
- Database Administrator – MS SQL/T-SQL/Azure SQL/SSMS
- Android App Development with Kotlin | Intermediate Android
- Python Projects: Python & Data Science with Python Projects
- Introduction to Server Driven UI in iOS, Swift & SwiftUI
- Master Visual Scripting in Unity by Making Advanced Games
- SQL for beginners with: Microsoft SQL Server | PostgreSQL