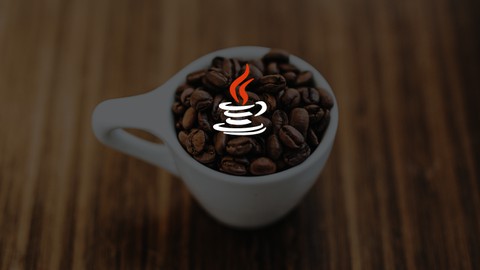
Programming Fundamentals in Java for CS Students: Part 1
Programming Fundamentals in Java for CS Students: Part 1, available at $19.99, has an average rating of 4.55, with 129 lectures, 6 quizzes, based on 37 reviews, and has 1209 subscribers.
You will learn about Develop a deep understanding of core programming concepts, including data structures, algorithms, and fundamental principles of programming. Learn how to write clean, efficient code using best practices, ensuring your code is easy to read, maintain, and optimize. Study key Java concepts such as variables, loops, conditionals, arrays, and functions that apply to other programming languages Understand how to apply the principles learned in this course to other programming languages, making you a more versatile and adaptable programmer. Gain confidence in explaining complex programming concepts to others, enhancing your communication skills and professional credibility. This course is ideal for individuals who are Computer Science students looking to strengthen their understanding of core programming concepts. or Aspiring software developers who want to learn Java and apply best practices in their coding. or Professionals seeking to improve their coding skills and learn how to write clean, efficient code. It is particularly useful for Computer Science students looking to strengthen their understanding of core programming concepts. or Aspiring software developers who want to learn Java and apply best practices in their coding. or Professionals seeking to improve their coding skills and learn how to write clean, efficient code.
Enroll now: Programming Fundamentals in Java for CS Students: Part 1
Summary
Title: Programming Fundamentals in Java for CS Students: Part 1
Price: $19.99
Average Rating: 4.55
Number of Lectures: 129
Number of Quizzes: 6
Number of Published Lectures: 129
Number of Published Quizzes: 6
Number of Curriculum Items: 138
Number of Published Curriculum Objects: 138
Original Price: $19.99
Quality Status: approved
Status: Live
What You Will Learn
- Develop a deep understanding of core programming concepts, including data structures, algorithms, and fundamental principles of programming.
- Learn how to write clean, efficient code using best practices, ensuring your code is easy to read, maintain, and optimize.
- Study key Java concepts such as variables, loops, conditionals, arrays, and functions that apply to other programming languages
- Understand how to apply the principles learned in this course to other programming languages, making you a more versatile and adaptable programmer.
- Gain confidence in explaining complex programming concepts to others, enhancing your communication skills and professional credibility.
Who Should Attend
- Computer Science students looking to strengthen their understanding of core programming concepts.
- Aspiring software developers who want to learn Java and apply best practices in their coding.
- Professionals seeking to improve their coding skills and learn how to write clean, efficient code.
Target Audiences
- Computer Science students looking to strengthen their understanding of core programming concepts.
- Aspiring software developers who want to learn Java and apply best practices in their coding.
- Professionals seeking to improve their coding skills and learn how to write clean, efficient code.
Welcome to “Programming Fundamentals in Java for CS Students“, an in-depth and comprehensive online course designed to provide you with a deep understanding of programming fundamentals using Java. This course will equip you with the skills to apply these concepts to other programming languages while emphasizing the importance of clean code and best practices.
Many online courses teach you how to perform tasks without building a strong foundation in programming concepts, leading to students who can write code but struggle to explain or optimize their work. This course aims to solve that problem by focusing on solid programming fundamentals, best practices, and clean code principles.
Target Audience:
This course is perfect for:
-
Computer Science students looking to strengthen their understanding of core programming concepts.
-
Aspiring software developers who want to learn Java and apply best practices in their coding.
Course Outline:
In this extensive course, you will:
-
Develop a deep understanding of core programming concepts.
-
Learn how to write clean, efficient code using best practices, ensuring your code is easy to read, maintain, and optimize.
-
Understand how to apply the principles learned in this course to other programming languages, making you a more versatile and adaptable programmer.
-
Gain confidencein explaining complex programming concepts to others, enhancing your communication skills and professional credibility.
-
Work through real-world examples and practice exercisesdesigned to reinforce your learning and prepare you for real-life programming challenges.
-
Study key Java concepts such as variables, loops, conditionals, arrays, and functions.
-
Participate in interactive coding challenges and projects to test your knowledge and sharpen your skills.
-
Benefit from instructor support and a vibrant community of fellow learners to enhance your learning experience.
By the end of this course, you will have a strong foundation in programming and will be well-equipped to write clean, efficient code in Javaand other programming languages. Join ustoday and start your journey towards becoming a skilled and confident programmer.
For those who are new to programming, this course offers an excellent starting point with its focus on the fundamentals. The course also includes a part II that delves into object-oriented programming. Once you feel comfortable with the basics, you can take advantage of my other course to explore object-oriented programming in greater depth and with greater confidence.
In addition to the core course content, you will also receive:
-
Access to exclusive, downloadable resources and cheat sheets for easy reference.
-
Lifetime access to course updates, ensuring you stay current with the latest Java developments and industry best practices.
-
A course completion certificate, demonstrating your commitment to mastering programming fundamentals and enhancing your professional credentials.
Enroll now and unlock your full potential in the world of programming! Don’t miss this opportunity to build a solid foundation in Java and other programming languages, and become the programmer you’ve always wanted to be.
Course Curriculum
Chapter 1: Introduction and environment setup
Lecture 1: Introduction
Lecture 2: Environment Setup
Lecture 3: New project and JDK installation
Lecture 4: Alternative solution
Chapter 2: Variables and basic data types
Lecture 1: Variables introduction
Lecture 2: Interactions between Hard disk, RAM and CPU
Lecture 3: Visual illustration of CPU RAM and Hard Drive Interactions
Lecture 4: Variables declaration and storage
Lecture 5: Code translation
Lecture 6: More about variables declaration
Lecture 7: Important: Java variables naming convention
Chapter 3: Data types
Lecture 1: Working with integers
Lecture 2: Code from the previous video
Lecture 3: Summary of the previous lecture
Lecture 4: Theory about floating-point data types
Lecture 5: Floating-point data types in practice
Lecture 6: Code from the previous video
Lecture 7: Working with texts and characters
Lecture 8: Strings and chars in practice
Lecture 9: Code from the previous video
Lecture 10: Quick discussion about booleans and Object types
Lecture 11: Comments in Java
Lecture 12: Section Summary
Lecture 13: Notes on the JVM
Chapter 4: Basic operations on data types
Lecture 1: The rectangle problem
Lecture 2: Code from previous lecture
Lecture 3: Difference between assignment and equality
Lecture 4: Code from previous lecture
Chapter 5: Displaying values
Lecture 1: Starter code for the next lecture
Lecture 2: Displaying numbers
Lecture 3: Code from the previous lecture
Lecture 4: Displaying numbers and type conversion
Lecture 5: Code from the previous lecture
Lecture 6: Displaying strings
Lecture 7: Code from the previous lecture
Lecture 8: Strings immutability
Lecture 9: Code from the previous lecture
Lecture 10: More about String immutability in Java
Lecture 11: Strings interpolation
Lecture 12: Code from the previous lecture
Lecture 13: More about printf() formatting
Lecture 14: Section summary
Chapter 6: Keyboard Input
Lecture 1: Accepting user inputs
Lecture 2: Code from the previous lecture
Lecture 3: Practice keyboard input
Lecture 4: Code from the previous lecture
Lecture 5: More on user inputs
Lecture 6: Code from the previous lecture
Lecture 7: How the next() function works internally
Lecture 8: Code from the previous lecture
Lecture 9: Reading an entire line of input with nextLine()
Lecture 10: Code from the previous lecture
Lecture 11: How nextInt() and nextDouble() work internally
Lecture 12: Code from the previous lecture
Lecture 13: Reading single characters from the keyboard
Lecture 14: Code form the previous lecture
Lecture 15: Section summary
Lecture 16: Note on closing the Scanner object
Chapter 7: Conditions
Lecture 1: Introduction
Lecture 2: Conditions explained with flow chart
Lecture 3: First program using conditions
Lecture 4: Code from the previous lecture
Lecture 5: Simple alternative
Lecture 6: Code from the previous lecture
Lecture 7: Boolean data type
Lecture 8: Code from the previous lecture
Lecture 9: Understanding conditions and Logical operator OR
Lecture 10: Logical operator OR in practice
Lecture 11: Code from the previous lecture
Lecture 12: Logical operator AND
Lecture 13: Code from the previous lecture
Lecture 14: Multiple alternatives
Lecture 15: Code from the previous lecture
Lecture 16: Ignoring case in string comparisons
Lecture 17: Code from the last lecture
Lecture 18: Modulo operator in Java
Chapter 8: Loops
Lecture 1: Code for the next lecture
Lecture 2: Loops : The problem
Lecture 3: For loop
Lecture 4: Code from the previous lecture
Lecture 5: In-depth analysis of how the for loop works
Lecture 6: For loop exercice
Lecture 7: Code from the previous lecture
Lecture 8: Do-while loop
Instructors
-
Christian Lisangola
Software Engineer & Trainer
Rating Distribution
- 1 stars: 0 votes
- 2 stars: 0 votes
- 3 stars: 2 votes
- 4 stars: 3 votes
- 5 stars: 32 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple