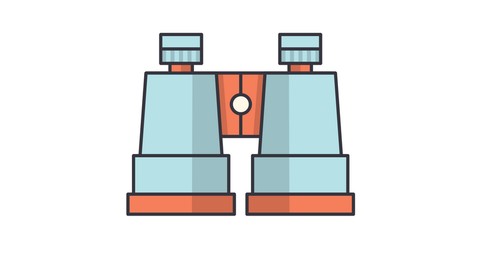
React Master Class – Learn By Coding Components
React Master Class – Learn By Coding Components, available at $69.99, has an average rating of 4.65, with 131 lectures, based on 108 reviews, and has 844 subscribers.
You will learn about Understand how setState works Understand common design patterns for building reusable components Better practices and learning's from the field Understand functional components and stateful components Understand how React works internally and code a basic version of React like library Build reusable React components of medium to high complexity. Build your own state management libary Understand Server Side Rendering This course is ideal for individuals who are Anyone who is tired of building a todo application and want to learn how to create a reusable medium complexity component and application should find this course interesting. It is particularly useful for Anyone who is tired of building a todo application and want to learn how to create a reusable medium complexity component and application should find this course interesting.
Enroll now: React Master Class – Learn By Coding Components
Summary
Title: React Master Class – Learn By Coding Components
Price: $69.99
Average Rating: 4.65
Number of Lectures: 131
Number of Published Lectures: 130
Number of Curriculum Items: 131
Number of Published Curriculum Objects: 130
Original Price: $199.99
Quality Status: approved
Status: Live
What You Will Learn
- Understand how setState works
- Understand common design patterns for building reusable components
- Better practices and learning's from the field
- Understand functional components and stateful components
- Understand how React works internally and code a basic version of React like library
- Build reusable React components of medium to high complexity.
- Build your own state management libary
- Understand Server Side Rendering
Who Should Attend
- Anyone who is tired of building a todo application and want to learn how to create a reusable medium complexity component and application should find this course interesting.
Target Audiences
- Anyone who is tired of building a todo application and want to learn how to create a reusable medium complexity component and application should find this course interesting.
Treat this offer as a “One Time Investment” to all future updates.
This course will take you from React Novice to React intermediate (advanced, or Expert level, with practice). We will covering some fundamentals in the beginning but move on to applications and the cool stuff.
In this course we will also code a reusable DataTable component from scratch, implement drag and drop without using any third-party library, create JSON based dynamic forms and much more.
You will learn many fundamental aspects of React Component design and also learn how to take this approach to build any UI component that you wish. Once you successfully complete the course you will have learned how to build a pretty advanced React component that contains the following features.
[ x ] React fundamentals
[ x ] Component in depth
[ x ] Hooks
[ x ] Understanding Virtual DOM and diffing
[ x ] Context API
[ x ] Learn to build dynamic form component using JSON.
[ x ] Customizing components using props
[ x ] Learn to add drag and drop feature without using third party library.
[ x ] Bind data to a table layout
[ x ] Searching on any columns
[ x ] Pagination, with custom pagination view
[ x ] Drag and reorder table columns
[ x ] In-place editing
[ x ] Custom cell renderer
[ x ] Attach the dynamic form to the DataTable.
[ x ] React Common Patterns
[ x ] State Management
[ x ] Server Side Rendering(SSR) and more
Planned overall agenda for the course:
Section 1 –
Fundamentals of React
-
Introduction
-
Fundamentals of React
-
JSX in depth
-
Component based design
-
Fragment
-
Functional Component
-
Stateful Component
-
Event Handling
-
Conditional
-
State Management
-
Life Cycle
-
Error Handling
Section 2 –
Advanced React
-
Type checking with PropTypes
-
Refs and the DOM
-
Uncontrolled Components
-
Higher Order Components (HOC)
-
Render Props
-
Performance Optimization
-
Context API
-
Portals
-
Integrating with other JavaScript
Section 3 – Building a simple CRUD app with React
Section 4 – New React Features
Section 5 – Context API Demo
Section 6 – Building a simple Modal Component
Section 7 – Building a Tagging Component
Section 8 – Building a Calendar Component
Section 9 – Building a DataTable Component
Section 10 – React Drag and Drop Tutorial
Bonus Section: (Dec 2018)
-
Server Side Rendering
We will minimize the use of third party libraries/frameworks and will only include them when absolutely required.
This course will be part of the Full Stack Engineering Open Source Curriculum and publishing on Udemy will kind of support the development of this.
NOTE: Most of the components developed in this training, is kept simple so that even a beginner could understand. To make it work in production scenarios, this has to be thoroughly tested and adapted as needed. I will keep on updating the content with better practices once the core course is completed.
Enjoy Programming!!
(Everyone can code!)
Course Curriculum
Chapter 1: Introduction
Lecture 1: Introduction to React curriculum
Lecture 2: Read this first please
Lecture 3: DataTable component overview
Lecture 4: Introduction to Calendar Component
Lecture 5: Introduction to React
Lecture 6: Create nested element using API
Chapter 2: Understanding JSX (Updated)
Lecture 1: Introduction to JSX
Lecture 2: JSX built in elements vs custom components
Lecture 3: Embedding Expressions in JSX
Lecture 4: Return multiple element from render
Chapter 3: Functional Components (Refer the later sections for changes in FC)
Lecture 1: Read me first please!
Lecture 2: Introduction to components
Lecture 3: Functional Component nesting/composition
Lecture 4: Using inline styles/variables within functional component
Chapter 4: Stateful or Class Component (KB for migration to newer React)
Lecture 1: Introduction to Stateful component
Lecture 2: A quick note on import statement
Lecture 3: Creating the App Component
Lecture 4: Introduction to State
Lecture 5: Creating Initial State
Lecture 6: Rendering Project List
Lecture 7: Component Design Introduction
Lecture 8: Extracting Project List to Component
Lecture 9: Refactoring – Extracting Props
Lecture 10: Add project to State
Lecture 11: Updating state with hardcoded object
Lecture 12: Destructuring Explanation
Chapter 5: How setState works (Updated)
Lecture 1: How setState works?
Chapter 6: CRUD Application (Updated)
Lecture 1: Creating a form for adding new Project
Lecture 2: Adding title field to form
Lecture 3: Adding select field to form
Lecture 4: Adding submit button to form
Lecture 5: Adding submit handler
Lecture 6: Accessing form values by using ref
Lecture 7: Adding new project to the app state and proptypes
Lecture 8: Edit Project
Lecture 9: Delete Project
Lecture 10: Mark a Project as completed
Chapter 7: Installing Create-React-App
Lecture 1: Setting up create-react-app
Lecture 2: Overview of files created by create-react-app
Chapter 8: Build a Dynamic JSON based Form Component
Lecture 1: Introduction
Lecture 2: Setting up the basic code structure
Lecture 3: Creating the DynamicForm Component Class
Lecture 4: Implementing the render method of DynamicForm
Lecture 5: Hooking on the submit event handler
Lecture 6: Setting up the loop to render form elements dynamically
Lecture 7: Rendering the labels dynamically
Lecture 8: Rendering form elements dynamically
Lecture 9: Adding support for Checkbox, Radio Buttons and Select Elements
Lecture 10: Dynamic form – bug fixes, updates and conclusion
Lecture 11: Notes on getDerivedStateFrom Props fix
Chapter 9: React Drag and Drop Tutorial (Updated)
Lecture 1: Setting up code
Lecture 2: Introduction
Lecture 3: What we will be building?
Lecture 4: The basic code to set things up and render the required user interface.
Lecture 5: Implementing the drag and drop feature
Chapter 10: Creating an InputTag component (Updated)
Lecture 1: Lets get coding the InputTag component
Chapter 11: Creating a Modal Component (Updated)
Lecture 1: Introduction
Lecture 2: Coding the modal component
Lecture 3: Setting Prop types
Lecture 4: Close modal on ESC key press
Lecture 5: React Portal Feature
Chapter 12: Creating a Calendar Component (Updated)
Lecture 1: Coding a calendar component – part -1
Lecture 2: Coding a calendar component – part -2
Chapter 13: React Context API
Lecture 1: Read Me First Please!
Lecture 2: Understanding the new Context API
Lecture 3: Some bug fixes
Lecture 4: Building a reusable state library using the new context API.
Chapter 14: HOC- Simple Example-withBorder
Lecture 1: Introduction to HOC
Lecture 2: Building the App component
Lecture 3: Implementing the withBorder HOC Component
Lecture 4: Refactor the withBorder HOC Component
Chapter 15: HOC- Intermediate Example-withCollapsible
Lecture 1: Coding a collapsible HOC component
Chapter 16: HOC – React Modal Dialog (Updated)
Lecture 1: Coding the React Modal Dialog as an HOC
Chapter 17: Render Props
Lecture 1: Render props
Chapter 18: Building your own DataTable Component
Lecture 1: Setting up basic code for DataTable
Lecture 2: Setting up the data for headers
Lecture 3: Setting up the table rows data
Lecture 4: Configuring DataTable in App Component
Lecture 5: Rendering the headers in the DataTable component
Lecture 6: Rendering the table body with data
Lecture 7: Sorting columns by clicking on the column headers
Lecture 8: Reordering columns/headers by drag and drop
Instructors
-
Rajesh Pillai
Founder at Algorisys Technologies and TekAcademy Labs
Rating Distribution
- 1 stars: 5 votes
- 2 stars: 2 votes
- 3 stars: 10 votes
- 4 stars: 28 votes
- 5 stars: 63 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple