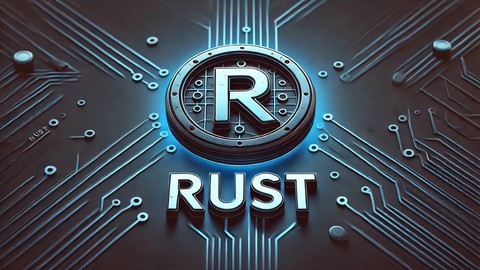
Rust: The Complete Developer's Guide
Rust: The Complete Developer's Guide, available at $54.99, has an average rating of 4.67, with 121 lectures, 11 quizzes, based on 255 reviews, and has 3211 subscribers.
You will learn about Master Rust's unique ownership system and borrowing rules for efficient memory management Understand and implement Rust's powerful enum types and pattern matching Develop proficiency in creating and organizing Rust projects using modules Learn to handle errors effectively using the Result type and various error-handling techniques Gain expertise in working with Rust's iterator system for efficient data processing Explore advanced lifetime concepts to manage complex memory relationships Implement generic types and traits to write flexible, reusable code Understand the differences between various Rust data structures like arrays, vectors, and slices Learn to work with external crates to extend your Rust programs' functionality Develop practical skills through hands-on projects and exercises, including file I/O operations This course is ideal for individuals who are Engineers looking to learn the basics of Rust It is particularly useful for Engineers looking to learn the basics of Rust.
Enroll now: Rust: The Complete Developer's Guide
Summary
Title: Rust: The Complete Developer's Guide
Price: $54.99
Average Rating: 4.67
Number of Lectures: 121
Number of Quizzes: 11
Number of Published Lectures: 121
Number of Published Quizzes: 11
Number of Curriculum Items: 132
Number of Published Curriculum Objects: 132
Original Price: $99.99
Quality Status: approved
Status: Live
What You Will Learn
- Master Rust's unique ownership system and borrowing rules for efficient memory management
- Understand and implement Rust's powerful enum types and pattern matching
- Develop proficiency in creating and organizing Rust projects using modules
- Learn to handle errors effectively using the Result type and various error-handling techniques
- Gain expertise in working with Rust's iterator system for efficient data processing
- Explore advanced lifetime concepts to manage complex memory relationships
- Implement generic types and traits to write flexible, reusable code
- Understand the differences between various Rust data structures like arrays, vectors, and slices
- Learn to work with external crates to extend your Rust programs' functionality
- Develop practical skills through hands-on projects and exercises, including file I/O operations
Who Should Attend
- Engineers looking to learn the basics of Rust
Target Audiences
- Engineers looking to learn the basics of Rust
Welcome to the most comprehensive and hands-on course for learning Rust from the ground up!
Rust is revolutionizing systems programming with its focus on memory safety, concurrency, and performance. But with its unique concepts and syntax, many find Rust challenging to learn. That’s where this course comes in – providing you with a clear, structured path to Rust mastery.
What sets this course apart? We focus on building a rock-solid foundation in Rust’s core concepts. No fluff, no skipping steps – just pure, essential Rust knowledge that will set you up for success in any Rust project.
Rust’s most challenging concepts are covered:
-
Rust’s ownership model? Explained in great detail!
-
Lifetimes and borrowing? Its here!
-
Traits and generics? You’ll use them to write flexible code
This course is designed for developers who want to truly understand Rust, not just copy-paste code. Whether you’re coming from Javascript, Python, or any other language, you’ll find a welcoming introduction to Rust’s unique paradigms.
Rust has been voted the “most loved programming language” in the Stack Overflow Developer Survey for seven consecutive years. It’s not just hype – major companies like Microsoft, Google, and Amazon are increasingly adopting Rust for critical systems. By mastering Rust, you’re not just learning a language; you’re future-proofing your career.
Here’s a (partial) list of what you’ll learn:
-
Dive deep into Rust’s type system and how it ensures memory safety
-
Master pattern matching and destructuring for elegant, expressive code
-
Harness the power of Rust’s error handling with Result and Option types
-
Explore Rust’s module system to organize and scale your projects
-
Implement common data structures and algorithms the Rust way
-
Use cargo to manage dependencies and build your projects with ease
-
A solid grasp of Rust’s syntax and core concepts
-
The ability to write safe, efficient, and idiomatic Rust code
-
Confidence to tackle real-world Rust projects and contribute to the ecosystem
-
A deep and fundamental understanding of error handling
-
The skills to optimize code for performance and memory usage
-
And much more!
How This Course Works:
This isn’t just another “follow along” coding course. We’ve structured the learning experience to ensure you truly internalize Rust’s concepts:
-
Concept Introduction: Clear, concise explanations of each Rust feature
-
Live Coding: Watch as we implement concepts in real-time, explaining our thought process
-
Challenges: Test your understanding with carefully crafted coding exercises
-
Project Work: Apply your skills to build progressively complex projects
-
Best Practices: Learn idiomatic Rust and industry-standard coding patterns
This is the course I wish I had when I was learning Rust. A course that focuses on the hardest parts, gives clear explanations, and discusses the pros and cons of different design options. Sign up today and join me in mastering Rust!
Course Curriculum
Chapter 1: Foundations of Rust: Setup and First Steps
Lecture 1: Introduction
Lecture 2: Rust Installation
Lecture 3: Local Rust Install
Lecture 4: Creating and Running Rust Projects
Lecture 5: Disabling Inlay Type Hints
Lecture 6: Course Resources
Chapter 2: Core Concepts: The Building Blocks of Rust
Lecture 1: Representing Data with Structs
Lecture 2: Adding Functionality to Structs
Lecture 3: Arrays vs Vectors
Lecture 4: Mutable vs Immutable Bindings
Lecture 5: Implementations and Methods
Lecture 6: Implicit Returns
Lecture 7: Installing External Crates
Lecture 8: Using Code from Crates
Lecture 9: Shuffling a Slice
Lecture 10: Splitting a Vector
Lecture 11: Project Review
Chapter 3: Ownership and Borrowing: Rust's Unique Memory System
Lecture 1: Project Overview
Lecture 2: Defining Structs
Lecture 3: Adding Inherent Implementations
Lecture 4: A Mysterious Error
Lecture 5: Unexpected Value Updates
Lecture 6: The Goal of Ownership and Borrowing
Lecture 7: The Basics of Ownership
Lecture 8: Visualizing Ownership and Moves
Lecture 9: Exercise Overview
Lecture 10: Exercise Solution
Lecture 11: Another Quick Exercise
Lecture 12: A Quick Exercise Solution
Lecture 13: Writing Useful Code with Ownership
Lecture 14: Introducing the Borrow System
Lecture 15: Immutable References
Lecture 16: Exercise On References
Lecture 17: References Exercise Solution
Lecture 18: Mutable References
Lecture 19: Exercise on Mutable Refs
Lecture 20: Solution on Mutable Refs
Lecture 21: Copy-able Values
Chapter 4: Lifetimes Explored: Understanding Memory Management
Lecture 1: Basics of Lifetimes
Lecture 2: Deciding on Argument Types
Lecture 3: Back to the Bank Impl
Lecture 4: Implementing Deposits and Withdrawals
Lecture 5: Accounts and Bank Implementation
Lecture 6: Project Wrapup
Chapter 5: Enums Unleashed: Pattern Matching and Options
Lecture 1: Project Overview
Lecture 2: Defining Enums
Lecture 3: Declaring Enum Values
Lecture 4: Adding Implementations to Enums
Lecture 5: Pattern Matching with Enums
Lecture 6: When to Use Structs vs Enums
Lecture 7: Adding Catalog Items
Lecture 8: Unlabeled Fields
Lecture 9: The Option Enum
Lecture 10: Option From Another Perspective
Lecture 11: Replacing Our Custom Enum with Option
Lecture 12: Other Ways of Handling Options
Lecture 13: Excercise Overview
Lecture 14: Exercise Solution
Chapter 6: Project Architecture: Mastering Modules in Rust
Lecture 1: Modules Overview
Lecture 2: Rules of Modules
Lecture 3: Refactoring with Multiple Modules
Chapter 7: Handling the Unexpected: Errors and Results
Lecture 1: Project Overview
Lecture 2: Reading a File
Lecture 3: The Result Enum
Lecture 4: The Result Enum in Action
Lecture 5: Types of Errors
Lecture 6: Matching on Results
Lecture 7: Empty OK Variants
Lecture 8: Exercise Around the Result Enum
Lecture 9: Exercise Solution
Lecture 10: Using a Result When Reading Files
Lecture 11: Tricky Strings
Lecture 12: The Stack and Heap
Lecture 13: Strings, String Refs, and String Slices
Lecture 14: When to Use Which String
Lecture 15: Finding Error Logs
Lecture 16: Understanding the Issue
Lecture 17: Fixing Errors Around String Slices
Lecture 18: Writing Data to a File
Lecture 19: Alternatives to Nested Matches
Lecture 20: The Try Operator
Lecture 21: When to Use Each Technique
Instructors
-
Stephen Grider
Engineering Architect
Rating Distribution
- 1 stars: 2 votes
- 2 stars: 4 votes
- 3 stars: 15 votes
- 4 stars: 57 votes
- 5 stars: 177 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple