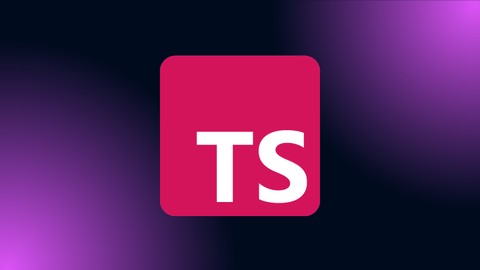
TypeScript Design Patterns And SOLID Principles
TypeScript Design Patterns And SOLID Principles, available at $19.99, has an average rating of 4.67, with 145 lectures, 26 quizzes, based on 222 reviews, and has 2125 subscribers.
You will learn about Master Gang of Four design patterns in TypeScript to write scalable and maintainable code for real-world applications. Gain in-depth understanding of SOLID principles to develop clean, modular, and robust TypeScript code for better software design. Creational Design Patterns including Singleton, Factory Method, Abstract Factory, Builder, and Prototype to effectively manage object creation in TypeScript. Gain expertise in Structural Design Patterns like Adapter, Bridge, Composite, Decorator and Facade to optimize your code. Behavioral Design Patterns including Observer, Strategy, Command, Iterator, State, Chain of Responsibility, and Visitor to enhance code flexibility. Grasp essential Object-Oriented Programming concepts such as inheritance, encapsulation, polymorphism, and abstraction to write clean, modular TypeScript code. Apply your knowledge through comprehensive real-world examples, solidifying your grasp on design patterns and OOP concepts in practical TypeScript projects. This course is ideal for individuals who are TypeScript developers eager to elevate their programming capabilities by mastering Design Patterns and SOLID principles. or Ideal for programmers intending to work on large-scale TypeScript projects, offering essential skills for maintainable and scalable code. or Perfect for TypeScript developers aiming to contribute to popular OOP-based GitHub repositories, providing the design pattern expertise required. or Ideal for those seeking to improve their ability to read and understand complex code, by mastering design patterns and OOP concepts. or Perfect for TypeScript developers aiming to elevate code quality through the effective use of design patterns and SOLID principles. It is particularly useful for TypeScript developers eager to elevate their programming capabilities by mastering Design Patterns and SOLID principles. or Ideal for programmers intending to work on large-scale TypeScript projects, offering essential skills for maintainable and scalable code. or Perfect for TypeScript developers aiming to contribute to popular OOP-based GitHub repositories, providing the design pattern expertise required. or Ideal for those seeking to improve their ability to read and understand complex code, by mastering design patterns and OOP concepts. or Perfect for TypeScript developers aiming to elevate code quality through the effective use of design patterns and SOLID principles.
Enroll now: TypeScript Design Patterns And SOLID Principles
Summary
Title: TypeScript Design Patterns And SOLID Principles
Price: $19.99
Average Rating: 4.67
Number of Lectures: 145
Number of Quizzes: 26
Number of Published Lectures: 145
Number of Published Quizzes: 26
Number of Curriculum Items: 171
Number of Published Curriculum Objects: 171
Original Price: $94.99
Quality Status: approved
Status: Live
What You Will Learn
- Master Gang of Four design patterns in TypeScript to write scalable and maintainable code for real-world applications.
- Gain in-depth understanding of SOLID principles to develop clean, modular, and robust TypeScript code for better software design.
- Creational Design Patterns including Singleton, Factory Method, Abstract Factory, Builder, and Prototype to effectively manage object creation in TypeScript.
- Gain expertise in Structural Design Patterns like Adapter, Bridge, Composite, Decorator and Facade to optimize your code.
- Behavioral Design Patterns including Observer, Strategy, Command, Iterator, State, Chain of Responsibility, and Visitor to enhance code flexibility.
- Grasp essential Object-Oriented Programming concepts such as inheritance, encapsulation, polymorphism, and abstraction to write clean, modular TypeScript code.
- Apply your knowledge through comprehensive real-world examples, solidifying your grasp on design patterns and OOP concepts in practical TypeScript projects.
Who Should Attend
- TypeScript developers eager to elevate their programming capabilities by mastering Design Patterns and SOLID principles.
- Ideal for programmers intending to work on large-scale TypeScript projects, offering essential skills for maintainable and scalable code.
- Perfect for TypeScript developers aiming to contribute to popular OOP-based GitHub repositories, providing the design pattern expertise required.
- Ideal for those seeking to improve their ability to read and understand complex code, by mastering design patterns and OOP concepts.
- Perfect for TypeScript developers aiming to elevate code quality through the effective use of design patterns and SOLID principles.
Target Audiences
- TypeScript developers eager to elevate their programming capabilities by mastering Design Patterns and SOLID principles.
- Ideal for programmers intending to work on large-scale TypeScript projects, offering essential skills for maintainable and scalable code.
- Perfect for TypeScript developers aiming to contribute to popular OOP-based GitHub repositories, providing the design pattern expertise required.
- Ideal for those seeking to improve their ability to read and understand complex code, by mastering design patterns and OOP concepts.
- Perfect for TypeScript developers aiming to elevate code quality through the effective use of design patterns and SOLID principles.
Welcome to this one-of-a-kind course specifically designed to transform your TypeScript programming skills by diving deep into the world of Gang Of Four Design Patterns, SOLID Design principles, and Object-Oriented Programming (OOP)concepts. Are you an aspiring or intermediate programmer looking to level up your game? Or are you an advanced programmer and need a refresher on the Gang Of Four Design Patterns and SOLID Design Principles? Do you have a grasp of TypeScript and now want to focus on architectural excellence and code reusability? If so, you’ve come to the right place!
This course isn’t just another tutorial; it’s your passport to becoming an advanced TypeScript developer. Throughout more than 140 high-definition videos, totaling over 10 hours of content, we’ll delve into the nuances of effective software design and programming. We go beyond theory by providing practical, hands-on coding exercises and quizzes that reinforce your learning and provide the skills you need for the real world. With this course, you don’t just learn; you practice, implement, and master the art of writing clean, efficient, and robust TypeScript code using the SOLID Design Principles and Gang Of For Design Patterns using TypeScript.
Uniquely, this course covers all three key areas you need for excellence in modern software development:
-
Design Patterns: Master the Gang Of Four Design Patterns like Singleton, Builder, Strategy, and many more to solve specific problems efficiently.
-
SOLID Design Principles: Understand and implement the SOLID principles that serve as the foundation for writing maintainable and scalable code.
-
Object-Oriented Programming Concepts: Learn and apply the four pillars of OOP—Inheritance, Encapsulation, Polymorphism, and Abstraction—in TypeScript, enabling you to write code that is both functional and elegant.
Design Patterns You Will Learn In This Course:
-
Creational Design Patterns
-
Factory
-
Abstract Factory
-
Builder
-
Prototype
-
Singleton
-
-
Structural Design Patterns
-
Decorator
-
Adapter
-
Facade
-
Bridge
-
Composite
-
-
Behavioral Design Patterns
-
Command
-
Chain of Responsibility
-
Observer Pattern
-
Interpreter
-
Iterator
-
State
-
Strategy
-
Template
-
By the end of this course, you’ll not only have a deep understanding of Software Design Patterns, SOLID principles, and OOP in TypeScript but also be equipped with the practical skills to apply these concepts in your future projects. Whether you are developing enterprise-level applications or working on freelance gigs, the skills you acquire here will make you stand out in the TypeScript development community.
Course Curriculum
Chapter 1: Intro To Design Patterns
Lecture 1: Welcome
Lecture 2: How To Make The Most Out Of This Course
Lecture 3: Note About Resource
Lecture 4: Intro To Design Patterns and Their History
Lecture 5: Why We Need Design Patterns
Lecture 6: Cautions and Considerations
Lecture 7: Classification Of Design Patterns
Lecture 8: Intro To UML
Lecture 9: Setup Development Environment
Chapter 2: OOP In TypeScript
Lecture 1: What is OOP
Lecture 2: OOP Lingo
Lecture 3: Abstraction
Lecture 4: Abstraction Implementation
Lecture 5: Abstraction Real World Use case
Lecture 6: Abstraction Advantages
Lecture 7: Encapsulation
Lecture 8: Encapsulation Implementation
Lecture 9: Encapsulation Advantages
Lecture 10: Polymorphism (Subtype)
Lecture 11: Polymorphism Use Cases
Lecture 12: Polymorphism Advantages
Lecture 13: Inheritance
Lecture 14: Inheritance Implementation
Lecture 15: Inheritance Advantages
Chapter 3: SOLID Design Principles
Lecture 1: SOLID Design Principles Introduction
Lecture 2: Single Responsibility Principle (SRP) Intro
Lecture 3: Real World Application Of SRP
Lecture 4: Advantages Single Responsibility Principle
Lecture 5: Open Closed Principle (OCP) Intro
Lecture 6: Real World Application Open Closed Principle
Lecture 7: Advantages Of Open Closed Principle
Lecture 8: Liskov Substitution Principle (LSP)
Lecture 9: Real World Application LSP
Lecture 10: Advantages Of The Liskov Substitution Principle
Lecture 11: Interface Segregation Principle (ISP)
Lecture 12: Real World Application of ISP
Lecture 13: Advantages Of Interface Segregation Principle
Lecture 14: Dependency Inversion Principle (DIP)
Lecture 15: Implementation Of Dependency Inversion Principle (DIP)
Lecture 16: Advantages Of Dependency Inversion Principle
Chapter 4: Creational Design Patterns
Lecture 1: Introduction To Creational Design Patterns
Lecture 2: Singleton Pattern
Lecture 3: When To Use Singleton Pattern
Lecture 4: Singleton Real World Implementation
Lecture 5: Singleton Advantages
Lecture 6: Caveats or Criticism Of Singleton Pattern
Lecture 7: Singleton Use Cases
Lecture 8: Prototype Pattern
Lecture 9: When To Use Prototype Pattern
Lecture 10: Prototype Real World Implementation
Lecture 11: Prototype Advantages
Lecture 12: Caveats or Criticism Of Prototype Pattern
Lecture 13: Prototype Use Cases
Lecture 14: Builder Pattern
Lecture 15: When To Use Builder Pattern
Lecture 16: Builder Real World Implementation
Lecture 17: Builder Pattern Advantages
Lecture 18: Caveats or Criticism Of Builder Pattern
Lecture 19: Builder Use Cases
Lecture 20: Factory Pattern
Lecture 21: When To Use Factory Pattern
Lecture 22: Factory Real World Implementation
Lecture 23: Factory Pattern Advantages
Lecture 24: Caveats or Criticism Of Factory Pattern
Lecture 25: Factory Pattern Use Cases
Lecture 26: Abstract Factory Pattern
Lecture 27: When To Use Abstract Factory Pattern
Lecture 28: Abstract Factory Real World Implementation
Lecture 29: Abstract Factory Advantages
Lecture 30: Caveats or Criticism Of Abstract Factory Pattern
Lecture 31: Abstract Factory Pattern Use Cases
Chapter 5: Structural Design Patterns
Lecture 1: Introduction To Structural Design Patterns
Lecture 2: Facade Pattern
Lecture 3: When To Use Facade Pattern
Lecture 4: Facade Real World Implementation
Lecture 5: Facade Pattern Advantages
Lecture 6: Caveats or Criticism Of Facade Pattern
Lecture 7: Facade Pattern Use Cases
Lecture 8: Bridge Pattern
Lecture 9: When To Use Bridge Pattern
Instructors
-
Manik (Cloudaffle)
Passionate Teacher | YouTuber | Full Stack Developer
Rating Distribution
- 1 stars: 2 votes
- 2 stars: 3 votes
- 3 stars: 16 votes
- 4 stars: 57 votes
- 5 stars: 145 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- Digital Marketing Foundation Course
- Google Shopping Ads Digital Marketing Course
- Multi Cloud Infrastructure for beginners
- Master Lead Generation: Grow Subscribers & Sales with Popups
- Complete Copywriting System : write to sell with ease
- Product Positioning Masterclass: Unlock Market Traction
- How to Promote Your Webinar and Get More Attendees?
- Digital Marketing Courses
- Create music with Artificial Intelligence in this new market
- Create CONVERTING UGC Content So Brands Will Pay You More
- Podcast: The top 8 ways to monetize by Podcasting
- TikTok Marketing Mastery: Learn to Grow & Go Viral
- Free Digital Marketing Basics Course in Hindi
- MailChimp Free Mailing Lists: MailChimp Email Marketing
- Automate Digital Marketing & Social Media with Generative AI
- Google Ads MasterClass – All Advanced Features
- Online Course Creator: Create & Sell Online Courses Today!
- Introduction to SEO – Basic Principles of SEO
- Affiliate Marketing For Beginners: Go From Novice To Pro
- Effective Website Planning Made Simple