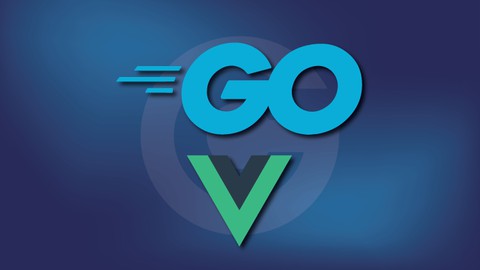
Working with Vue 3 and Go (Golang)
Working with Vue 3 and Go (Golang), available at $69.99, has an average rating of 4.62, with 152 lectures, based on 315 reviews, and has 2705 subscribers.
You will learn about Learn how to create interactive Web applications using Vue 3 Learn how to create a REST backend using Go (often referred to as Golang) Learn how to create a secure user authentication system with Vue and Go Learn best practices for creating a secure, scalable web application This course is ideal for individuals who are This course is intended for developers who have some experience with JavaScript, Go, and HTML It is particularly useful for This course is intended for developers who have some experience with JavaScript, Go, and HTML.
Enroll now: Working with Vue 3 and Go (Golang)
Summary
Title: Working with Vue 3 and Go (Golang)
Price: $69.99
Average Rating: 4.62
Number of Lectures: 152
Number of Published Lectures: 152
Number of Curriculum Items: 152
Number of Published Curriculum Objects: 152
Original Price: $69.99
Quality Status: approved
Status: Live
What You Will Learn
- Learn how to create interactive Web applications using Vue 3
- Learn how to create a REST backend using Go (often referred to as Golang)
- Learn how to create a secure user authentication system with Vue and Go
- Learn best practices for creating a secure, scalable web application
Who Should Attend
- This course is intended for developers who have some experience with JavaScript, Go, and HTML
Target Audiences
- This course is intended for developers who have some experience with JavaScript, Go, and HTML
Vue.js is, as they say on their website, an “approachable, performant and versatile framework for building web user interfaces.” That sentence really does not give Vue its full due. It is arguably the best solution currently available for building highly interactive, easy to maintain, and feature-rich web applications currently available. Many developers find it easier to learn than React or Angular, and once you learn the basics, it is easy to move on to building more complex applications.
Go, commonly referred to as Golang, is an easy to learn, type safe, compiled programming language that has quickly become a favourite among people writing API back ends, network software, and similar products. The ease with which Go works with JSON, for example, makes it an ideal solution to develop a back end for a Single Page Application written in something like Vue.
This course will cover all of the things you need to know to start writing feature-rich, highly interactive applications using Vue.js version 3 for the front end, and Go for the back end API.
We will cover:
-
Working with Vue 3 using a CDN
-
Working with Vue 3 using the vue-cli, node.js and npm
-
Learn both the Options API and the Composition API for Vue 3
-
How to work with props
-
How to build reusable Vue components
-
How to build and use a data store with Vue 3
-
Creating, validating, and posting forms using fetch and JSON
-
Emitting and processing events in Vue
-
Conditional rendering in Vue
-
Animations and transitions in Vue
-
Working with the Vue Router
-
Protecting routes in Vue (requiring user authentication)
-
Caching components using Vue’s KeepAlive functionality
-
Implementing a REST API using Go
-
Routing with Go
-
Connecting to a Postgresql database with Go
-
Reading and writing JSON with Go
-
Complete user authentication with Go using stateful tokens
-
Testing our Go back end with unit and integration tests
-
And much more.
Vue is one of the most popular front end JavaScript frameworks out there, and Go is quickly becoming the must-know language for developers, so learning them is definitely a benefit for any developer.
Course Curriculum
Chapter 1: Introduction
Lecture 1: Introduction
Lecture 2: About me
Lecture 3: Options API vs. Composition API
Lecture 4: Installing Go
Lecture 5: Installing Visual Studio Code
Lecture 6: Installing the Vetur VS Code extension
Lecture 7: Installing make
Lecture 8: How to ask for help
Chapter 2: Getting Started with Vue
Lecture 1: What we'll cover in this section
Lecture 2: Using Vue with a CDN ( we'll use npm later)
Lecture 3: The structure of a Vue app
Lecture 4: Adding a simple form element and binding data
Lecture 5: Adding a counter
Lecture 6: Vue Components – Getting Started
Lecture 7: Creating a reusable form input component
Lecture 8: Trying out our TextInput component
Lecture 9: The Vue lifecycle and client side validation
Lecture 10: Nesting components within components
Lecture 11: Adding a select form component
Lecture 12: Adding a checkbox form component
Lecture 13: Conditional Rendering
Lecture 14: Fetching remote data
Lecture 15: Using the data we fetch in our Vue application
Lecture 16: Adding some interactivity to our list of books
Lecture 17: Removing a book from the list
Chapter 3: Working with Vue using Single File Components, the vue-cli and Node.js
Lecture 1: Installing Node.js
Lecture 2: Installing vue-cli
Lecture 3: Building and running a simple Vue application with vue-cli
Lecture 4: The structure of a vue-cli application
Lecture 5: Getting started with our app
Lecture 6: Registering header, body, and footer components
Lecture 7: Adding navigation to our header component
Lecture 8: Adding content to our body component
Lecture 9: Adding content to our footer component
Chapter 4: Routing with the Vue Router
Lecture 1: Installing the Vue Router
Lecture 2: Getting started with the Vue Router
Lecture 3: Adding our routes in App.vue and main.js
Lecture 4: Creating a second component and updating our navigation links
Lecture 5: Adding and using our form Vue Components from the previous section
Lecture 6: Creating and implementing a re-usable Form tag component
Lecture 7: Improving our Login form by binding data and listening for an event
Lecture 8: Improving our FormTag and simplifying client side validation
Chapter 5: Building a Go REST back end
Lecture 1: How Go works with JSON files
Lecture 2: Setting up our project
Lecture 3: Improving routes
Lecture 4: Connecting from Vue to Go – the first try
Lecture 5: Implementing CORS in our routes.go file
Lecture 6: Connecting from Vue to Go – the second try
Lecture 7: ReadJSON and WriteJSON helper functions
Lecture 8: Simplifying our handlers to use the new helper functions
Lecture 9: Adding a helper function to generate error messages
Chapter 6: Authentication
Lecture 1: Setting up a database with Docker
Lecture 2: Choosing a Postgres client
Lecture 3: Trying out Beekeeper and setting up a users table
Lecture 4: Creating a database driver package
Lecture 5: Connecting to the database using our driver package
Lecture 6: Using a Makefile to simplify our lives
Lecture 7: Setting up a User model
Lecture 8: Setting up the tokens table
Lecture 9: Setting up a Token model
Lecture 10: How we will write database functions for authentication
Lecture 11: Manually adding a user to the database
Lecture 12: Creating a test route and handler to try things out
Lecture 13: Writing methods to get a user by email or id
Lecture 14: Writing methods to update and delete users
Lecture 15: Writing the Insert method for the User type
Lecture 16: Writing the Password reset and PasswordMatches functions for the User type
Lecture 17: Getting started with methods for the Token type
Lecture 18: Generating and authenticating a token
Lecture 19: Inserting and deleting tokens
Lecture 20: Adding a ValidToken method to the Token type
Lecture 21: Trying out the database functions: adding a user
Lecture 22: Trying out generating a token
Lecture 23: Trying out the Token type's Insert function
Lecture 24: Trying out the ValidToken function
Lecture 25: Adding a unique constraint to the users table
Lecture 26: Checking for database errors in errorJSON
Lecture 27: Improving our jsonResponse type with an envelope
Chapter 7: Authentication from Vue to the Go Back End
Lecture 1: Updating our Login handler
Lecture 2: Updating the Vue front end to connect to /users/auth and get a token
Lecture 3: Trying out authentication
Lecture 4: Sharing data between components using a simple store
Lecture 5: Improving the login process
Lecture 6: Logging out
Lecture 7: Deleting a user's token on the back end on logout
Lecture 8: Making the request to delete a token from the front end
Lecture 9: Saving the token as a cookie
Lecture 10: Finishing up the improved login process
Lecture 11: An aside: speeding things up when writing JSON in production
Chapter 8: Protecting Routes and managing site content
Lecture 1: Protecting routes
Lecture 2: Trying out our route protection middleware
Lecture 3: Setting up stub components and routes
Instructors
-
Trevor Sawler
Ph.D.
Rating Distribution
- 1 stars: 3 votes
- 2 stars: 6 votes
- 3 stars: 10 votes
- 4 stars: 50 votes
- 5 stars: 246 votes
Frequently Asked Questions
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don’t have an internet connection, some instructors also let their students download course lectures. That’s up to the instructor though, so make sure you get on their good side!
You may also like
- ISO 9001:2015 Quality Management System (QMS)
- Start Your Own Business: From Startup Idea to Product Launch
- Agile, Scrum & Kanban Practice Tests & Interview Questions
- Should I Start a Home Care Agency?
- Customer Segmentation Analytics Masterclass 2024
- Amazon FBA&Dropshipping Suspension Prevention Course (2023)
- How To Finance Deals With Little To No Money Out Of Pocket
- Transport and Mobility Decarbonisation & Energy Transition
- Project Management Professional (PMP) 6th edition PMBOK
- Building Better Programs: The Training Cycle
- Professional Email Writing in English: Complete Training
- Create & Sell – Finding your IDEAL CUSTOMER
- ESG Reporting
- FOOD SAFETY Exam Questions Practice Test
- PMI's Disciplined Agile Coach- Practice Exam
- Business the Hard Way Vol 1: Sales the Hard Way
- Using & Interpreting CamelCamelCamel & Keepa Charts For FBA
- Brand Identity Breakthrough: Make Your Products Irresistible
- Webinar Mastery – Sell High Ticket Products!
- How to Draft Privacy policy & Terms of Use for your website